Java将HTML转化为PDF+获得页数+合并PDF
时间: 2023-07-31 16:13:31 浏览: 112
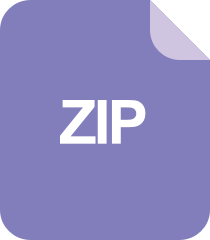
Java实现将html转PDF

好的,我来回答你的问题。
1. Java将HTML转化为PDF
你可以使用开源的工具,比如 iText、Flying Saucer、PDFBox 等来实现将 HTML 转化为 PDF 的功能。其中,iText 是比较常用的一个工具,你可以通过以下代码实现将 HTML 转化为 PDF:
```java
import com.itextpdf.html2pdf.HtmlConverter;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class HtmlToPdf {
public static void main(String[] args) throws IOException {
FileInputStream htmlFile = new FileInputStream("input.html");
FileOutputStream pdfFile = new FileOutputStream("output.pdf");
HtmlConverter.convertToPdf(htmlFile, pdfFile);
}
}
```
2. 获得页数
使用 iText 工具,你可以通过以下代码获得 PDF 文件的页数:
```java
import com.itextpdf.text.pdf.PdfReader;
public class GetPageCount {
public static void main(String[] args) throws IOException {
PdfReader pdfReader = new PdfReader("input.pdf");
int pageCount = pdfReader.getNumberOfPages();
System.out.println("Page count: " + pageCount);
pdfReader.close();
}
}
```
3. 合并PDF
使用 iText 工具,你可以通过以下代码实现合并多个 PDF 文件:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.PdfCopy;
import com.itextpdf.text.pdf.PdfReader;
import java.io.FileOutputStream;
import java.io.IOException;
public class MergePdf {
public static void main(String[] args) throws IOException {
Document document = new Document();
PdfCopy copy = new PdfCopy(document, new FileOutputStream("output.pdf"));
document.open();
PdfReader reader1 = new PdfReader("input1.pdf");
PdfReader reader2 = new PdfReader("input2.pdf");
copy.addDocument(reader1);
copy.addDocument(reader2);
document.close();
reader1.close();
reader2.close();
}
}
```
希望这些代码能对你有所帮助!
阅读全文
相关推荐
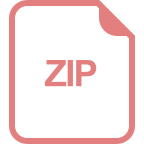

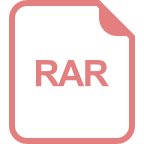
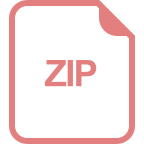
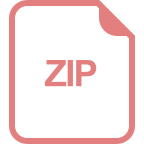
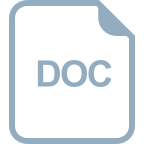
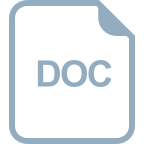
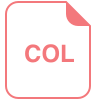
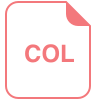


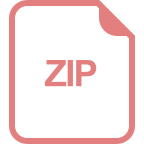
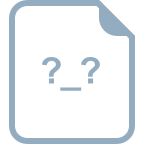
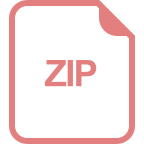
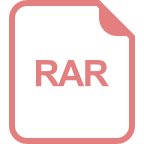
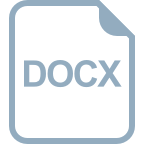
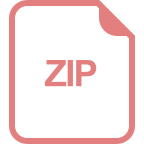