Java tcp NIO发送接收例子
时间: 2023-07-29 07:10:52 浏览: 65
以下是一个使用Java NIO实现TCP发送和接收的例子:
```java
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
public class NioTcpClient {
private static final String SERVER_IP = "localhost";
private static final int SERVER_PORT = 8888;
public static void main(String[] args) throws Exception {
SocketChannel socketChannel = SocketChannel.open();
socketChannel.configureBlocking(false);
socketChannel.connect(new InetSocketAddress(SERVER_IP, SERVER_PORT));
// 等待连接完成
while (!socketChannel.finishConnect()) {
Thread.sleep(100);
}
// 发送数据
String message = "Hello, Server!";
ByteBuffer buffer = ByteBuffer.wrap(message.getBytes());
socketChannel.write(buffer);
// 接收数据
buffer.clear();
int bytesRead = socketChannel.read(buffer);
if (bytesRead > 0) {
buffer.flip();
byte[] bytes = new byte[buffer.remaining()];
buffer.get(bytes);
String response = new String(bytes, "UTF-8");
System.out.println("Received message from server: " + response);
}
socketChannel.close();
}
}
```
在这个例子中,首先创建一个SocketChannel实例,然后设置为非阻塞模式,并通过connect()方法连接到服务器。由于非阻塞模式下连接是异步的,因此需要通过循环等待连接完成。连接完成后,可以通过write()方法发送数据,通过read()方法接收数据。需要注意的是,在非阻塞模式下,这些方法的返回值不一定是完整的数据,因此需要通过ByteBuffer进行缓冲和处理。最后,关闭SocketChannel实例。
相关推荐
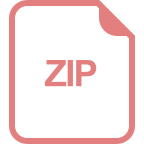














