用java写一个批量交换机巡检工具并输出到文件中
时间: 2024-03-01 17:54:25 浏览: 85
好的,下面是一个用Java写的批量交换机巡检工具的示例代码,并将输出保存到文件中:
```java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import com.jcraft.jsch.Channel;
import com.jcraft.jsch.ChannelExec;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.JSchException;
import com.jcraft.jsch.Session;
public class SwitchInspector {
// 定义交换机列表,每个元素是一个数组,包含交换机的IP地址、用户名和密码
private static final String[][] SWITCHES = {
{"192.168.1.1", "admin", "password"},
{"192.168.1.2", "admin", "password"},
{"192.168.1.3", "admin", "password"}
};
// 定义需要执行的命令列表
private static final String[] COMMANDS = {
"show version",
"show interface status",
"show interfaces gigabitEthernet 1/0/1",
"show ip interface brief"
};
public static void main(String[] args) {
for (String[] switchInfo : SWITCHES) {
String ip = switchInfo[0];
String username = switchInfo[1];
String password = switchInfo[2];
// 连接交换机并执行命令
try {
Session session = connect(ip, username, password);
List<String> outputList = new ArrayList<String>();
for (String command : COMMANDS) {
String output = executeCommand(session, command);
outputList.add(String.format("Output for command %s:\n%s\n", command, output));
}
saveOutputToFile(ip, outputList);
session.disconnect();
} catch (JSchException | IOException e) {
e.printStackTrace();
}
}
}
// 连接交换机
private static Session connect(String ip, String username, String password) throws JSchException {
JSch jsch = new JSch();
Session session = jsch.getSession(username, ip, 22);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
return session;
}
// 执行命令并返回输出
private static String executeCommand(Session session, String command) throws JSchException, IOException {
Channel channel = session.openChannel("exec");
((ChannelExec) channel).setCommand(command);
channel.setInputStream(null);
((ChannelExec) channel).setErrStream(System.err);
InputStream in = channel.getInputStream();
channel.connect();
StringBuilder output = new StringBuilder();
byte[] tmp = new byte[1024];
while (true) {
while (in.available() > 0) {
int i = in.read(tmp, 0, 1024);
if (i < 0) break;
output.append(new String(tmp, 0, i));
}
if (channel.isClosed()) {
if (in.available() > 0) continue;
break;
}
try { Thread.sleep(1000); } catch (Exception e) {}
}
channel.disconnect();
return output.toString();
}
// 将输出保存到文件中
private static void saveOutputToFile(String ip, List<String> outputList) throws IOException {
try (BufferedWriter writer = new BufferedWriter(new FileWriter(String.format("output_%s.txt", ip)))) {
for (String output : outputList) {
writer.write(output);
}
}
}
}
```
以上代码使用了JSch库来与交换机进行SSH连接,并执行命令。你需要替换代码中的交换机IP地址、用户名和密码,以及需要执行的命令列表。执行代码后,将会在当前目录下生成多个输出文件,每个文件对应一个交换机的输出。你可以将其修改为将输出保存到其他路径或其他格式的文件中。
阅读全文
相关推荐
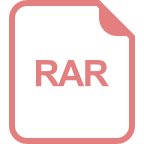


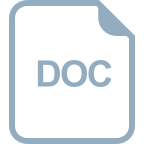
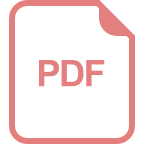
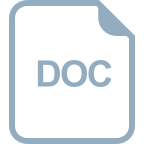
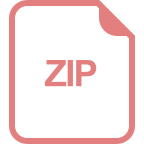
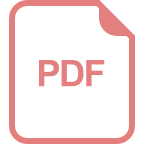
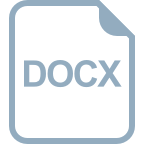
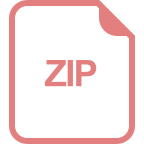
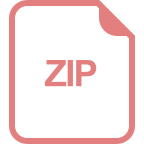
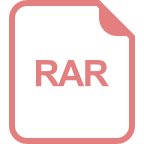
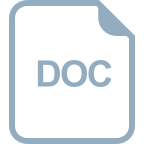