用C语言再写一个读入数据生成链表并处理的程序:⚫从上述input.ifo文件读入人员信息,正向建立线性表,使用循环链表方式存储;⚫输出线性表各结点的值和在线性表的位置值到屏幕;⚫键盘输入编号值S,在线性表中查找到编号为S的结点并输出结点的值,包括名字和随机编号(需要测试查找到和查找不到两种情况);⚫键盘输入位置值M,从线性表中1号结点开始向后报数,将报M的结点从线性表中删除,并输出该结点的编号和初始位置;(提示:可以将按位置删除函数改造成一个新的删除函数,删除的同时返回指向下一个结点的指针值、被删除结点的值;初始位置的值,可以考虑在建立循环链表时保存到结点内)⚫从刚才被删除人的下一个人开始重复上述步骤,直至最后只剩下一个人为止;⚫输出最后剩余一个人的编号和在线性表的初始位置。
时间: 2024-02-01 19:14:47 浏览: 219
以下是一个简单的实现,代码中有注释说明:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义一个人员信息结构体
typedef struct {
int id;
char name[20];
} Person;
// 定义一个循环链表结构体
typedef struct node {
Person data;
struct node *next;
} Node, *LinkedList;
// 读入人员信息并建立循环链表
LinkedList createList() {
FILE *fp = fopen("input.info", "r");
if (fp == NULL) {
printf("Fail to open file.");
exit(1);
}
LinkedList head = NULL, tail = NULL;
while (!feof(fp)) {
Person person;
fscanf(fp, "%d %s", &person.id, person.name);
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = person;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
tail->next = head; // 将尾结点的 next 指向头结点,形成循环链表
fclose(fp);
return head;
}
// 输出链表中所有结点的信息
void printList(LinkedList head) {
Node *p = head;
int i = 1;
do {
printf("Node %d: ID=%d, Name=%s\n", i++, p->data.id, p->data.name);
p = p->next;
} while (p != head);
}
// 根据编号查找结点
Node *findNodeById(LinkedList head, int id) {
Node *p = head;
do {
if (p->data.id == id) {
return p;
}
p = p->next;
} while (p != head);
return NULL;
}
// 根据位置删除结点并返回指向下一个结点的指针值、被删除结点的值和初始位置的值
Node *deleteNodeByPosition(LinkedList head, int pos, Person *deletedPerson, int *initialPos) {
Node *p = head, *prev = NULL;
int i = 1;
while (i < pos && p->next != head) {
prev = p;
p = p->next;
i++;
}
if (i < pos) { // 位置值超过了链表长度
return NULL;
}
if (prev == NULL) { // 删除头结点
prev = head;
while (prev->next != head) {
prev = prev->next;
}
head = head->next;
prev->next = head;
} else {
prev->next = p->next;
}
*deletedPerson = p->data;
*initialPos = i;
free(p);
return prev->next;
}
int main() {
LinkedList list = createList();
printf("The linked list is:\n");
printList(list);
int id;
printf("Please enter an ID to search: ");
scanf("%d", &id);
Node *node = findNodeById(list, id);
if (node == NULL) {
printf("No such node found.\n");
} else {
printf("Node with ID=%d and Name=%s is found at position %d.\n", node->data.id, node->data.name, (int)(node - list) + 1);
}
int pos;
printf("Please enter a position to delete: ");
scanf("%d", &pos);
Person deletedPerson;
int initialPos;
Node *nextNode = deleteNodeByPosition(list, pos, &deletedPerson, &initialPos);
if (nextNode == NULL) {
printf("Invalid position.\n");
} else {
printf("Node with ID=%d and Name=%s is deleted from position %d.\n", deletedPerson.id, deletedPerson.name, initialPos);
while (nextNode->next != nextNode) {
printf("The linked list is:\n");
printList(nextNode);
printf("Please enter a position to delete: ");
scanf("%d", &pos);
nextNode = deleteNodeByPosition(nextNode, pos, &deletedPerson, &initialPos);
printf("Node with ID=%d and Name=%s is deleted from position %d.\n", deletedPerson.id, deletedPerson.name, initialPos);
}
printf("The last person left is with ID=%d and is at the initial position %d.\n", nextNode->data.id, (int)(nextNode - list) + 1);
}
return 0;
}
```
阅读全文
相关推荐
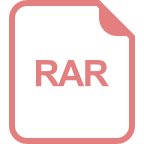




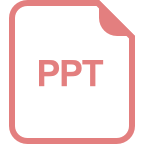
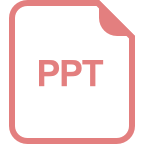
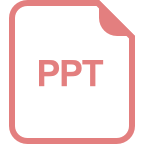
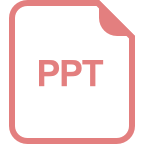
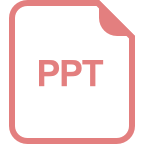
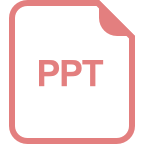
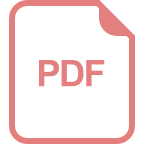
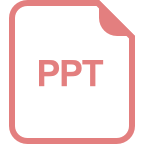
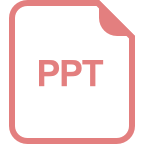
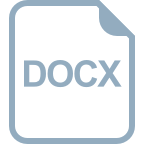
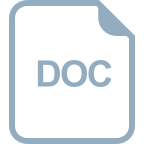