根据SSH私钥(private key)计算SSH公钥(public key)
时间: 2023-07-05 14:05:42 浏览: 72
在Linux或MacOS上,可以使用以下命令来生成SSH公钥:
```
ssh-keygen -y -f /path/to/private_key > /path/to/public_key
```
其中,`/path/to/private_key`是你的SSH私钥文件路径,`/path/to/public_key`是你要生成的SSH公钥文件路径。
该命令会从指定的私钥文件中读取私钥,然后计算出对应的公钥,并将公钥输出到指定的公钥文件中。你可以将生成的公钥文件发送给需要使用该公钥进行认证的人或系统。
相关问题
ssh公钥私钥换算器
SSH公钥和私钥是一对密钥,它们被用于在SSH协议下进行身份验证和加密通信。这里提供一个简单的SSH公钥和私钥换算器的Python代码示例,可以将一个格式正确的SSH私钥转换为公钥或者将公钥转换为私钥。
```python
import base64
import hashlib
def generate_public_key(private_key):
"""
从SSH私钥生成SSH公钥
"""
key_type, _, private_key = private_key.split(' ', 2)
private_key = base64.b64decode(private_key)
if key_type == 'ssh-rsa':
# RSA公钥格式: ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQ...
exponent_length = int.from_bytes(private_key[0:4], byteorder='big')
exponent = int.from_bytes(private_key[4:4+exponent_length], byteorder='big')
modulus_length = int.from_bytes(private_key[4+exponent_length:8+exponent_length], byteorder='big')
modulus = int.from_bytes(private_key[8+exponent_length:8+exponent_length+modulus_length], byteorder='big')
public_key = 'ssh-rsa ' + base64.b64encode(
b'\x00\x00\x00\x07ssh-rsa' + exponent_length.to_bytes(4, byteorder='big') + exponent.to_bytes((exponent.bit_length() + 7) // 8, byteorder='big') + modulus_length.to_bytes(4, byteorder='big') + modulus.to_bytes((modulus.bit_length() + 7) // 8, byteorder='big')
).decode('ascii')
return public_key
elif key_type == 'ssh-ed25519':
# Ed25519公钥格式: ssh-ed25519 AAAAC3NzaC1lZDI1NTE5AAAAIMcDa...
public_key = 'ssh-ed25519 ' + base64.b64encode(private_key[32:]).decode('ascii')
return public_key
else:
raise ValueError('Unsupported key type')
def generate_private_key(public_key, private_key=None):
"""
从SSH公钥生成SSH私钥
"""
key_type, _, public_key = public_key.split(' ', 2)
public_key = base64.b64decode(public_key)
if key_type == 'ssh-rsa':
# RSA私钥格式: ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQ...
if private_key is None:
raise ValueError('Private key is required for RSA key type')
exponent_length = private_key.find(b'\x00\x00\x00\x07ssh-rsa') + 11
exponent_length = int.from_bytes(private_key[exponent_length:exponent_length+4], byteorder='big')
exponent = int.from_bytes(private_key[exponent_length+4:exponent_length+4+exponent_length], byteorder='big')
modulus_length = int.from_bytes(private_key[exponent_length+4+exponent_length:exponent_length+4+exponent_length+4], byteorder='big')
modulus = int.from_bytes(private_key[exponent_length+4+exponent_length+4:exponent_length+4+exponent_length+4+modulus_length], byteorder='big')
private_key = b''.join([
b'\x00\x00\x00\x07ssh-rsa',
exponent_length.to_bytes(4, byteorder='big'),
exponent.to_bytes((exponent.bit_length() + 7) // 8, byteorder='big'),
modulus_length.to_bytes(4, byteorder='big'),
modulus.to_bytes((modulus.bit_length() + 7) // 8, byteorder='big'),
private_key[public_key.__len__():]
])
private_key = key_type.encode('ascii') + b' ' + base64.b64encode(private_key).replace(b'\n', b'') + b' '
return private_key
elif key_type == 'ssh-ed25519':
# Ed25519私钥格式: ssh-ed25519 AAAAC3NzaC1lZDI1NTE5AAAAIMcDa...
if private_key is None:
# 使用公钥生成随机私钥
private_key = hashlib.sha256(public_key).digest()
private_key = key_type.encode('ascii') + b' ' + base64.b64encode(private_key).replace(b'\n', b'') + b' '
return private_key
else:
raise ValueError('Unsupported key type')
```
使用示例:
```python
# 生成RSA密钥对
from cryptography.hazmat.primitives.asymmetric import rsa
private_key = rsa.generate_private_key(public_exponent=65537, key_size=2048)
public_key = private_key.public_key()
ssh_private_key = generate_private_key('ssh-rsa ' + base64.b64encode(public_key.public_bytes(encoding=serialization.Encoding.DER, format=serialization.PublicFormat.PKCS1)).decode('ascii'), private_key=private_key.private_bytes(encoding=serialization.Encoding.DER, format=serialization.PrivateFormat.PKCS8, encryption_algorithm=serialization.NoEncryption()))
ssh_public_key = generate_public_key(ssh_private_key)
# 生成Ed25519密钥对
from cryptography.hazmat.primitives.asymmetric import ed25519
private_key = ed25519.Ed25519PrivateKey.generate()
public_key = private_key.public_key()
ssh_private_key = generate_private_key('ssh-ed25519 ' + base64.b64encode(public_key.public_bytes(encoding=serialization.Encoding.Raw, format=serialization.PublicFormat.Raw)).decode('ascii'))
ssh_public_key = generate_public_key(ssh_private_key)
```
注意:这个示例代码仅适用于支持PKCS#1和PKCS#8格式的RSA和Ed25519密钥。如果要使用其他格式的密钥,代码需要进行相应的修改。此外,为了安全起见,不应该在生产环境中使用此代码,而应该使用已经被广泛测试和验证的SSH密钥生成库。
SSH public key
SSH public key是通过使用ssh-keygen命令生成的一种加密密钥对中的公钥。在使用ssh-keygen命令时,通过指定选项-t rsa,可以生成一对RSA算法的公钥和私钥。公钥可以被分享给其他人或在服务器上配置,用于进行安全的SSH连接验证。私钥则应该妥善保管,不应该被分享或泄露。
在Jenkins中,使用Publish over SSH插件时,可以将生成的公钥配置到服务器上,用于实现安全的SSH连接管理。服务器上存放的是公钥文件,而Jenkins上存放的是私钥文件,用于进行身份验证。通过这种方式,可以实现无密码连接服务器的操作。
总结来说,SSH public key是通过ssh-keygen命令生成的一种加密密钥对中的公钥,用于进行安全的SSH连接验证。可以将公钥配置到服务器上,实现无密码连接服务器的操作。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [ssh-keygen(linux 命令) 创建 private key(私钥) , public key (公钥),实现ssh,scp,sftp命令无密码...](https://blog.csdn.net/sxzlc/article/details/126356471)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [Xshell配置ssh免密码登录-密钥公钥(Public key)](https://blog.csdn.net/lgxzzz/article/details/124409658)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
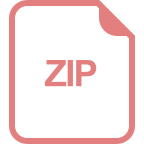
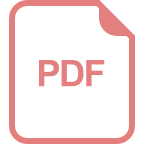
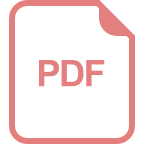












