用C语言统计二叉树中叶子结点数目,并输出二叉树中叶子结点数目,计算树的深度
时间: 2024-03-23 12:41:22 浏览: 103
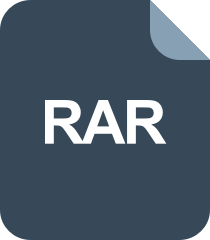
用C语言求二叉树叶子结点

好的,我来为你提供一个用 C 语言实现的统计二叉树中叶子节点数目和计算树的深度的代码。假设二叉树的节点结构体定义如下:
```c
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
```
统计叶子节点数目的代码如下:
```c
int countLeaves(struct TreeNode* root) {
if (!root) {
return 0;
}
if (!root->left && !root->right) {
return 1;
}
int leftLeaves = countLeaves(root->left);
int rightLeaves = countLeaves(root->right);
return leftLeaves + rightLeaves;
}
```
计算树的深度的代码如下:
```c
int maxDepth(struct TreeNode* root) {
if (!root) {
return 0;
}
if (!root->left && !root->right) {
return 1;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
```
注意,在 C 语言中,函数名不能包含大写字母。因此,我将原来的函数名 count_leaves 和 max_depth 改为了 countLeaves 和 maxDepth。如果需要使用原来的函数名,可以在定义函数时将函数名改为小写字母形式。
阅读全文
相关推荐















