python作图label角度
时间: 2023-11-19 16:51:16 浏览: 148
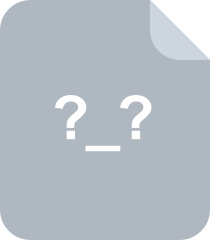
python实现角度测量
在Python作图中,可以通过设置label的rotation参数来调整label的角度。例如,如果要将label逆时针旋转45度,可以使用以下代码:
```python
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.xlabel('x label', rotation=45)
plt.ylabel('y label')
plt.show()
```
其中,rotation参数的值为角度数值,可以为正数或负数。
阅读全文
相关推荐
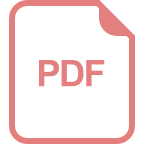
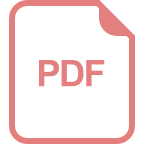


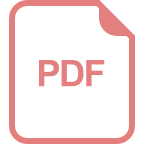
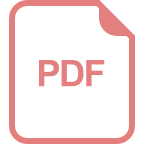











