c++ 实现json转bytes
时间: 2023-12-10 15:37:18 浏览: 28
以下是使用C++实现将JSON转换为字节数组的示例代码:
```c++
#include <iostream>
#include <string>
#include <fstream>
#include <sstream>
#include <vector>
#include "json.hpp"
using json = nlohmann::json;
int main()
{
// 读取JSON文件
std::ifstream ifs("example.json");
std::stringstream buffer;
buffer << ifs.rdbuf();
std::string json_str = buffer.str();
// 解析JSON
json j = json::parse(json_str);
// 将JSON转换为字节数组
std::vector<uint8_t> bytes;
for (auto& element : j.items()) {
std::string key = element.key();
json value = element.value();
// 处理不同类型的JSON值
if (value.is_string()) {
std::string str_value = value.get<std::string>();
bytes.insert(bytes.end(), str_value.begin(), str_value.end());
} else if (value.is_number()) {
int num_value = value.get<int>();
bytes.push_back(num_value);
} else if (value.is_boolean()) {
bool bool_value = value.get<bool>();
bytes.push_back(bool_value ? 1 : 0);
} else if (value.is_object() || value.is_array()) {
// 递归处理JSON对象或数组
std::stringstream ss;
ss << value;
std::string str_value = ss.str();
bytes.insert(bytes.end(), str_value.begin(), str_value.end());
}
}
// 输出字节数组
for (auto& byte : bytes) {
std::cout << std::hex << (int)byte << " ";
}
std::cout << std::endl;
return 0;
}
```
在这个示例中,我们使用nlohmann/json库来解析JSON字符串,并将JSON转换为字节数组。对于不同类型的JSON值,我们使用if语句进行处理。如果是字符串,我们将其转换为字节数组,如果是数字或布尔值,我们将其直接添加到字节数组中。如果是JSON对象或数组,我们递归地处理它们。最后,我们将字节数组输出到控制台。
需要注意的是,这只是一个简单的示例,可能无法处理所有类型的JSON值。在实际使用中,您可能需要根据具体的需求进行修改和扩展。
相关推荐











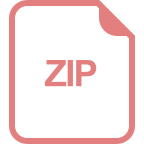
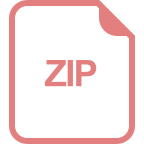