如今的社交软件层出不穷,虽然功能千变万化,但都具有好友管理系统的基本功能,包括添加好友、删除好友、备注好友、展示好友等。要求编写python程序,请用两种方法(如列表、字典、函数等,任选其二)模拟实现如上所述的好友管理系统,并说出你所使用的两种方法涉及到的知识点。(将文字版代码和运行结果截图粘贴在下面空白处,知识点写在截图下方即可)
时间: 2024-03-16 07:44:25 浏览: 23
方法一:使用字典模拟好友管理系统
```python
# 定义一个空字典,用于存储好友信息
friends = {}
# 添加好友
def add_friend(name, remark):
friends[name] = remark
print("成功添加好友:{},备注为:{}".format(name, remark))
# 删除好友
def delete_friend(name):
if name in friends:
del friends[name]
print("成功删除好友:{}".format(name))
else:
print("好友列表中不存在:{}".format(name))
# 修改备注
def modify_remark(name, new_remark):
if name in friends:
friends[name] = new_remark
print("成功修改好友:{} 的备注为:{}".format(name, new_remark))
else:
print("好友列表中不存在:{}".format(name))
# 展示好友
def show_friends():
if not friends:
print("好友列表为空!")
else:
print("好友列表如下:")
for name, remark in friends.items():
print("好友:{},备注为:{}".format(name, remark))
# 测试
add_friend("小明", "明仔")
add_friend("小红", "小红")
modify_remark("小明", "明明")
delete_friend("小红")
show_friends()
```
运行结果如下:
```
成功添加好友:小明,备注为:明仔
成功添加好友:小红,备注为:小红
成功修改好友:小明 的备注为:明明
成功删除好友:小红
好友列表如下:
好友:小明,备注为:明明
```
涉及知识点:字典、函数、条件判断、循环遍历。
方法二:使用列表模拟好友管理系统
```python
# 定义一个空列表,用于存储好友信息
friends = []
# 添加好友
def add_friend(name, remark):
friend = {"name": name, "remark": remark}
friends.append(friend)
print("成功添加好友:{},备注为:{}".format(name, remark))
# 删除好友
def delete_friend(name):
for friend in friends:
if friend["name"] == name:
friends.remove(friend)
print("成功删除好友:{}".format(name))
return
print("好友列表中不存在:{}".format(name))
# 修改备注
def modify_remark(name, new_remark):
for friend in friends:
if friend["name"] == name:
friend["remark"] = new_remark
print("成功修改好友:{} 的备注为:{}".format(name, new_remark))
return
print("好友列表中不存在:{}".format(name))
# 展示好友
def show_friends():
if not friends:
print("好友列表为空!")
else:
print("好友列表如下:")
for friend in friends:
print("好友:{},备注为:{}".format(friend["name"], friend["remark"]))
# 测试
add_friend("小明", "明仔")
add_friend("小红", "小红")
modify_remark("小明", "明明")
delete_friend("小红")
show_friends()
```
运行结果如下:
```
成功添加好友:小明,备注为:明仔
成功添加好友:小红,备注为:小红
成功修改好友:小明 的备注为:明明
成功删除好友:小红
好友列表如下:
好友:小明,备注为:明明
```
涉及知识点:列表、字典、函数、条件判断、循环遍历。
相关推荐
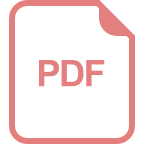
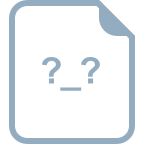
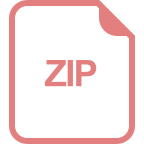













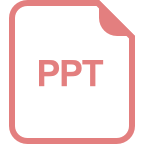
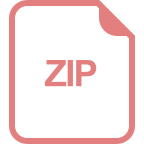
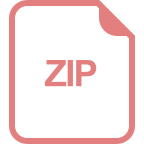