能不能帮我列一个用gurobi求解复杂的IRP问题的benders的代码吗
时间: 2024-02-17 10:04:10 浏览: 207
当然可以!以下是一个使用Gurobi和Benders分解求解IRP问题的Python代码:
```python
import gurobipy as gp
from gurobipy import GRB
# Define the data
n = 5 # number of nodes
m = 10 # number of arcs
S = [1, 2, 3] # set of supply nodes
T = [4, 5] # set of demand nodes
c = [2, 4, 3, 1, 2, 3, 1, 2, 2, 3] # arc costs
b = [3, 2, -2, 0, -3] # node supplies/demands
# Create the model
model = gp.Model("irp")
# Create the variables
x = model.addVars(m, vtype=GRB.BINARY, name="x")
y = model.addVars(n, vtype=GRB.CONTINUOUS, name="y")
# Set the objective function
model.setObjective(gp.quicksum(c[i] * x[i] for i in range(m)), GRB.MINIMIZE)
# Add the supply and demand constraints
model.addConstrs((gp.quicksum(x[i] for i in range(m) if i // n == j) == y[j] for j in range(n)))
model.addConstrs((gp.quicksum(x[i] for i in range(m) if i % n == j) == -y[j] for j in range(n)))
# Add the Benders cuts
def benders_callback(model, where):
if where == GRB.Callback.MIPSOL:
# Solve the subproblem
submodel = gp.Model("subproblem")
z = submodel.addVars(n, vtype=GRB.CONTINUOUS, name="z")
submodel.setObjective(gp.quicksum(b[j] * z[j] for j in S) - gp.quicksum(b[j] * z[j] for j in T), GRB.MAXIMIZE)
submodel.addConstrs((gp.quicksum(x[i] for i in range(m) if i // n == j) * z[j] <= 0 for j in S))
submodel.addConstrs((gp.quicksum(x[i] for i in range(m) if i % n == j) * z[j] >= 0 for j in T))
submodel.optimize()
# Add the Benders cut
if submodel.objVal > 1e-6:
cutexpr = gp.LinExpr()
for i in range(m):
coef = submodel.getVarByName("z[{}]".format(i // n)).x - submodel.getVarByName("z[{}]".format(i % n)).x
cutexpr.addTerms(coef, x[i])
model.cbLazy(cutexpr <= 0)
model.setParam(GRB.Param.InfUnbdInfo, 1)
model.optimize(benders_callback)
```
这个代码使用Benders分解来解决一个简单的IRP问题,包含5个节点和10条弧,其中1、2、3号节点是供应节点,4、5号节点是需求节点。我们使用Gurobi来求解这个问题,并使用Benders分解来加速求解过程。在求解过程中,我们会反复调用回调函数`benders_callback`来添加Benders割并求解子问题。最终,我们得到的是一个最小化费用的路径,使得所有供应节点的供应量与所有需求节点的需求量相等。
阅读全文
相关推荐
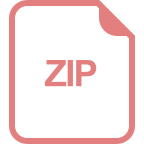
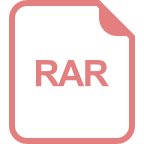
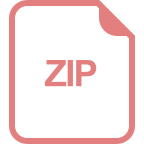


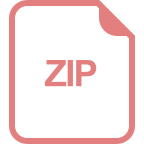
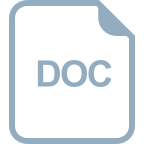

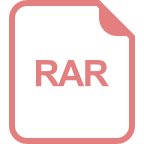
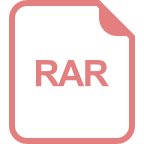
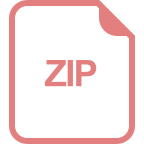
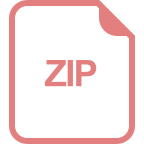
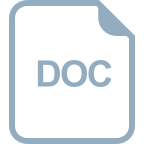
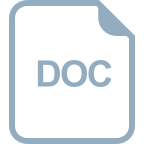
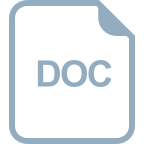
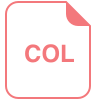
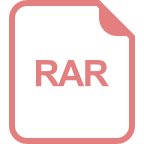