集合运算的C语言代码
时间: 2024-12-04 20:13:31 浏览: 12
在C语言中,集合运算主要包括并集(union)、交集(intersection),以及差集(difference)。这些操作通常用于数组或其他数据结构(如指针)上。
1. **并集(Union)**:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int element;
} Item;
Item* union_array(Item a[], Item b[], int n1, int n2) {
Item* result = (Item*)malloc((n1 + n2) * sizeof(Item));
int i = 0, j = 0;
while (i < n1 && j < n2) {
if (a[i].element < b[j].element) {
result[i] = a[i];
i++;
} else if (b[j].element < a[i].element) {
result[j] = b[j];
j++;
} else { // 如果相等,取任意一个放入结果,并移动相应的指针
result[i] = a[i]; // 或 result[j] = b[j];
i++; j++;
}
}
// 将剩余未复制的数据添加到结果数组
while (i < n1) {
result[j++] = a[i++];
}
while (j < n2) {
result[j++] = b[j - 1];
}
return result;
}
// 使用示例
int main() {
Item a[] = {1, 2, 3};
Item b[] = {2, 4, 5};
int n1 = sizeof(a) / sizeof(a[0]);
int n2 = sizeof(b) / sizeof(b[0]);
Item* union_result = union_array(a, b, n1, n2);
for (int i = 0; i < n1 + n2; i++) {
printf("%d ", union_result[i].element);
}
free(union_result);
return 0;
}
```
2. **交集(Intersection)**:实现这个功能相对复杂些,因为C标准库中没有提供直接的交集操作。你可以通过遍历两个集合并在新数组中只存储相同的元素来模拟:
```c
void intersection(Item* result, Item a[], Item b[], int n1, int n2) {
int index = 0;
for (int i = 0; i < n1 && index < n2; ++i) {
if (a[i].element == b[index]) {
result[index] = a[i];
index++;
}
}
result[index] = '\0'; // 结束符,表示已经处理完所有元素
}
```
注意,这里只是一个简单的示例,实际应用中可能会使用更高效的数据结构或算法。
3. **差集(Difference)**:同样,C标准库也没有提供直接的差集函数,可以手动创建一个函数来实现:
```c
void difference(Item* result, Item from[], Item to[], int n1, int n2) {
for (int i = 0; i < n1; ++i) {
bool found = false;
for (int j = 0; j < n2; ++j) {
if (from[i].element == to[j].element) {
found = true;
break;
}
}
if (!found) {
result[i] = from[i];
}
}
result[n1] = '\0';
}
```
阅读全文
相关推荐








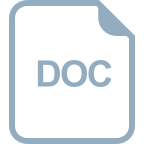
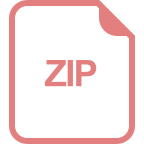





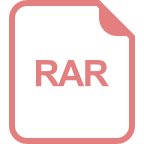

