用c语言编写程序实现统计一个字符串中各元音字母(即A,E,I,O,U)的个数。不区分大小写。 然后将统计的数据按序输出。 输入格式: asdfghjklmnopqrstuvwxyz 输出格式: Please enter a string: 1 0 0 1 1 说明:每个数字宽度为4,右对齐。 输入样例: asdfghjklmnopqrstuvwxyz 输出样例: Please enter a string: 1 0 0 1 1
时间: 2023-06-05 12:06:31 浏览: 1148
请按照以下方式编写程序:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int main()
{
char str[100];
int cnt[5] = {0}; // 分别统计 AEIOU 出现的次数
int i;
printf("Please enter a string: ");
scanf("%s", str);
for (i = 0; i < strlen(str); i++)
{
switch (tolower(str[i]))
{
case 'a': cnt[0]++; break;
case 'e': cnt[1]++; break;
case 'i': cnt[2]++; break;
case 'o': cnt[3]++; break;
case 'u': cnt[4]++; break;
}
}
printf("%4d %4d %4d %4d %4d\n", cnt[0], cnt[1], cnt[2], cnt[3], cnt[4]);
return 0;
}
```
注意事项:
- `str` 的长度不要超过 99。
- `tolower` 函数可以将大写字母转换为小写字母,避免了大小写造成的统计错误。
- 格式化输出时,数字占用 4 个字符宽度,右对齐。
相关问题
用C语言编写程序,统计字符串中元音字母的个数
下面是一个用 C 语言编写的统计字符串中元音字母个数的程序:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
int vowels = 0;
printf("请输入一个字符串:");
fgets(str, 100, stdin);
for (int i = 0; i < strlen(str); i++) {
switch (str[i]) {
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
case 'A':
case 'E':
case 'I':
case 'O':
case 'U':
vowels++;
break;
default:
break;
}
}
printf("元音字母的个数为:%d\n", vowels);
return 0;
}
```
这个程序首先提示用户输入一个字符串,然后使用 `fgets()` 函数读取输入的字符串。接下来,程序使用 `for` 循环遍历字符串中的每一个字符,并使用 `switch` 语句判断该字符是否为元音字母。如果是,则将元音字母计数器加1。最后,程序输出元音字母的个数。
编写一个C语言程序,统计并显示从键盘输入的字符串中元音字母'a’、e 、i、o’、"u’的个数
以下是一个简单的 C 语言程序,用于统计并显示从键盘输入的字符串中元音字母 'a'、'e'、'i'、'o'、'u' 的个数:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char str[100];
int len, i, count = 0;
printf("请输入一个字符串:");
gets(str);
len = strlen(str);
for (i = 0; i < len; i++) {
switch (str[i]) {
case 'a':
case 'A':
case 'e':
case 'E':
case 'i':
case 'I':
case 'o':
case 'O':
case 'u':
case 'U':
count++;
break;
default:
break;
}
}
printf("元音字母的个数是:%d\n", count);
return 0;
}
```
程序首先使用 `gets()` 函数从键盘读取一个字符串,然后使用 `strlen()` 函数计算字符串的长度。接下来,使用一个循环遍历字符串中的每个字符,如果该字符是元音字母之一,则将计数器加一。最后,输出元音字母的个数。注意,程序中使用了 `switch` 语句来判断字符是否为元音字母,同时考虑了大小写字母的情况。
相关推荐
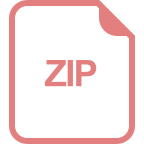
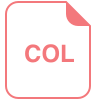
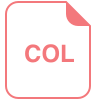
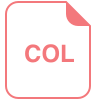
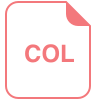
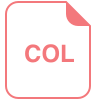





