vue判断input里的光标是否失去焦点
时间: 2023-07-26 19:39:12 浏览: 70
可以通过监听 `blur` 事件来判断输入框是否失去焦点。例如:
```html
<template>
<div>
<input type="text" v-model="message" @blur="handleBlur">
</div>
</template>
<script>
export default {
data() {
return {
message: ''
}
},
methods: {
handleBlur() {
console.log('输入框失去焦点了')
}
}
}
</script>
```
当输入框失去焦点时,`handleBlur` 方法会被触发,可以在此方法中执行你需要的逻辑。
相关问题
vue获取input光标位置
你可以使用 `document.activeElement` 来获取当前具有焦点的元素,然后使用该元素的 `selectionStart` 属性来获取光标位置。
以下是一个示例:
```html
<template>
<div>
<input ref="input" type="text" @input="handleInput">
<button @click="getCursorPosition">获取光标位置</button>
</div>
</template>
<script>
export default {
methods: {
handleInput() {
// 处理输入事件
},
getCursorPosition() {
const inputElement = this.$refs.input;
const cursorPosition = inputElement.selectionStart;
console.log('光标位置:', cursorPosition);
}
}
}
</script>
```
在上面的示例中,我们通过 `ref` 属性给 `<input>` 元素命名为 "input",然后在 `getCursorPosition` 方法中使用 `this.$refs.input` 来获取该元素的引用。然后,我们可以使用 `selectionStart` 属性获取光标的位置,并将其打印到控制台上。
希望对你有帮助!
antdesignvue select 获得焦点时 输入框获得光标
可以通过`ref`来获取`Select`组件的实例,然后在`focus`事件中调用输入框的`focus()`方法来让输入框获得光标。具体代码如下:
```vue
<template>
<a-select ref="mySelect" v-on:focus="handleFocus">
<!-- options -->
</a-select>
</template>
<script>
export default {
methods: {
handleFocus() {
this.$refs.mySelect.$refs.input.focus()
}
}
}
</script>
```
在`handleFocus`方法中,我们通过`this.$refs.mySelect`获取`Select`组件的实例,然后通过`$refs.input`获取输入框的实例,最后调用`focus()`方法让输入框获得光标。
相关推荐
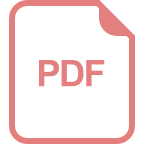












