winfrom 做一款书架,可以预览
时间: 2024-04-15 12:28:44 浏览: 58
你可以使用 WinForms 来开发一款书架应用程序,并在应用程序中实现书籍的预览功能。以下是一个简单的示例代码,用于创建一个基本的书架应用程序:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Windows.Forms;
namespace BookshelfApp
{
public partial class BookshelfForm : Form
{
private List<string> bookPaths;
public BookshelfForm()
{
InitializeComponent();
bookPaths = new List<string>();
}
private void addButton_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "文本文件 (*.txt)|*.txt|所有文件 (*.*)|*.*";
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
string bookPath = openFileDialog.FileName;
bookPaths.Add(bookPath);
bookListBox.Items.Add(Path.GetFileName(bookPath));
}
}
private void viewButton_Click(object sender, EventArgs e)
{
if (bookListBox.SelectedIndex >= 0)
{
string selectedBookPath = bookPaths[bookListBox.SelectedIndex];
string bookContent = File.ReadAllText(selectedBookPath);
PreviewForm previewForm = new PreviewForm(bookContent);
previewForm.ShowDialog();
}
}
}
public partial class PreviewForm : Form
{
public PreviewForm(string bookContent)
{
InitializeComponent();
previewTextBox.Text = bookContent;
}
}
}
```
在这个示例中,我们创建了一个 `BookshelfForm` 类来实现书架应用程序的主窗体。主窗体包含一个 "添加" 按钮和一个 "预览" 按钮。当用户点击 "添加" 按钮时,会弹出一个文件选择对话框,用户可以选择要添加到书架的书籍文件。选择文件后,文件路径会保存在 `bookPaths` 列表中,并在书架列表框中显示文件名。
当用户点击 "预览" 按钮时,会检查书架列表框中是否选择了一本书籍。如果选择了一本书籍,将获取选定书籍的路径,并读取该书籍的内容。然后,创建一个 `PreviewForm` 对象来显示书籍的预览内容。
你可以根据实际需求进行修改和扩展这个示例,比如添加更多的功能、美化界面等。
阅读全文
相关推荐
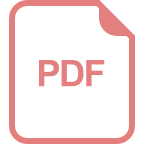
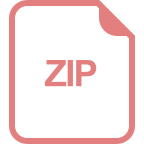
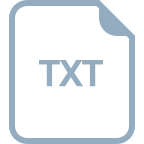
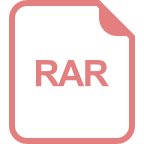
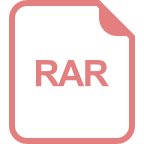
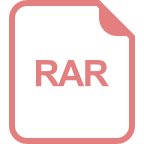
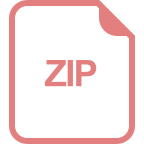
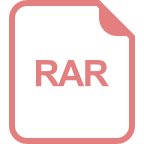
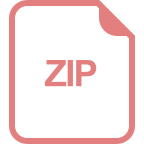
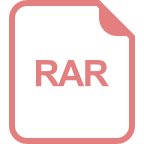
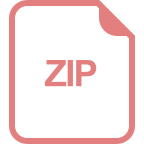
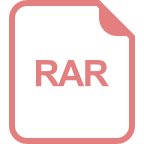
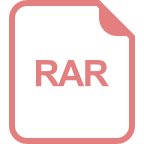
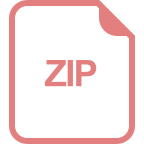
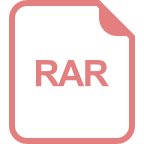
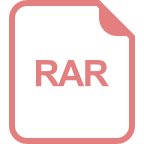
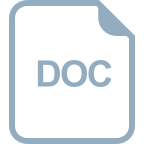