调用百度图像审核接口,使用Java语言再写3个示例代码
时间: 2024-06-09 21:07:26 浏览: 20
好的,以下是三个使用Java调用百度图像审核接口的示例代码:
1. 使用Java的HttpURLConnection实现:
```java
import java.io.*;
import java.net.*;
public class ImageAuditDemo1 {
public static void main(String[] args) throws Exception {
String url = "https://aip.baidubce.com/rest/2.0/solution/v1/img_censor/v2/user_defined";
String apiKey = "Your API Key";
String secretKey = "Your Secret Key";
String filePath = "image.jpg";
URL requestUrl = new URL(url + "?access_token=" + getAccessToken(apiKey, secretKey));
HttpURLConnection conn = (HttpURLConnection) requestUrl.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
conn.setDoOutput(true);
OutputStream outputStream = conn.getOutputStream();
FileInputStream fileInputStream = new FileInputStream(filePath);
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
fileInputStream.close();
outputStream.close();
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String line = null;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
}
private static String getAccessToken(String apiKey, String secretKey) {
try {
String authUrl = "https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id="
+ apiKey + "&client_secret=" + secretKey;
URL url = new URL(authUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String line = null;
StringBuilder sb = new StringBuilder();
while ((line = reader.readLine()) != null) {
sb.append(line);
}
reader.close();
String jsonStr = sb.toString();
int startIndex = jsonStr.indexOf("access_token") + "access_token".length() + 3;
int endIndex = jsonStr.indexOf(",", startIndex) - 1;
return jsonStr.substring(startIndex, endIndex);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
```
2. 使用Java的HttpClient实现:
```java
import java.io.*;
import org.apache.http.*;
import org.apache.http.client.*;
import org.apache.http.client.methods.*;
import org.apache.http.entity.mime.*;
import org.apache.http.entity.mime.content.*;
import org.apache.http.impl.client.*;
import org.apache.http.message.*;
public class ImageAuditDemo2 {
public static void main(String[] args) throws Exception {
String url = "https://aip.baidubce.com/rest/2.0/solution/v1/img_censor/v2/user_defined";
String apiKey = "Your API Key";
String secretKey = "Your Secret Key";
String filePath = "image.jpg";
String accessToken = getAccessToken(apiKey, secretKey);
HttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost(url + "?access_token=" + accessToken);
FileBody fileBody = new FileBody(new File(filePath));
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addPart("image", fileBody);
HttpEntity entity = builder.build();
httpPost.setEntity(entity);
HttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
BufferedReader reader = new BufferedReader(new InputStreamReader(responseEntity.getContent()));
StringBuilder sb = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
sb.append(line);
}
System.out.println(sb.toString());
}
private static String getAccessToken(String apiKey, String secretKey) {
try {
String authUrl = "https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id="
+ apiKey + "&client_secret=" + secretKey;
HttpClient httpClient = HttpClientBuilder.create().build();
HttpGet httpGet = new HttpGet(authUrl);
HttpResponse response = httpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
BufferedReader reader = new BufferedReader(new InputStreamReader(entity.getContent()));
StringBuilder sb = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
sb.append(line);
}
reader.close();
String jsonStr = sb.toString();
int startIndex = jsonStr.indexOf("access_token") + "access_token".length() + 3;
int endIndex = jsonStr.indexOf(",", startIndex) - 1;
return jsonStr.substring(startIndex, endIndex);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
```
3. 使用Java的OkHttp实现:
```java
import java.io.*;
import okhttp3.*;
public class ImageAuditDemo3 {
public static void main(String[] args) throws Exception {
String url = "https://aip.baidubce.com/rest/2.0/solution/v1/img_censor/v2/user_defined";
String apiKey = "Your API Key";
String secretKey = "Your Secret Key";
String filePath = "image.jpg";
String accessToken = getAccessToken(apiKey, secretKey);
OkHttpClient httpClient = new OkHttpClient();
RequestBody requestBody = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("image", "image.jpg", RequestBody.create(MediaType.parse("image/jpeg"), new File(filePath)))
.build();
Request request = new Request.Builder().url(url + "?access_token=" + accessToken).post(requestBody).build();
Response response = httpClient.newCall(request).execute();
String responseBody = response.body().string();
System.out.println(responseBody);
}
private static String getAccessToken(String apiKey, String secretKey) {
try {
String authUrl = "https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id="
+ apiKey + "&client_secret=" + secretKey;
OkHttpClient httpClient = new OkHttpClient();
Request request = new Request.Builder().url(authUrl).get().build();
Response response = httpClient.newCall(request).execute();
String responseBody = response.body().string();
int startIndex = responseBody.indexOf("access_token") + "access_token".length() + 3;
int endIndex = responseBody.indexOf(",", startIndex) - 1;
return responseBody.substring(startIndex, endIndex);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
```
需要注意的是,以上示例代码中的 `Your API Key` 和 `Your Secret Key` 应该替换成你在百度AI平台上申请的API Key和Secret Key。同时,示例代码中的图片路径也需要替换成你自己的图片路径。
相关推荐
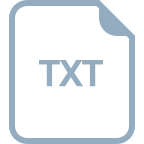
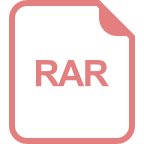
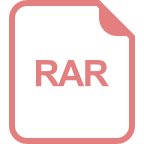















