csharp 读取和写入ini文件示例
时间: 2023-07-18 16:03:19 浏览: 65
下面是一个简单的示例,演示如何在C#中读取和写入INI文件:
读取INI文件:
```csharp
using System.Runtime.InteropServices;
using System.Text;
public class IniFile
{
private string path;
[DllImport("kernel32")]
private static extern long GetPrivateProfileString(string section, string key, string def, StringBuilder retVal, int size, string filePath);
public IniFile(string INIPath)
{
path = INIPath;
}
public string Read(string section, string key)
{
StringBuilder retVal = new StringBuilder(255);
long i = GetPrivateProfileString(section, key, "", retVal, 255, path);
return retVal.ToString();
}
}
```
写入INI文件:
```csharp
using System.Runtime.InteropServices;
public class IniFile
{
private string path;
[DllImport("kernel32")]
private static extern long WritePrivateProfileString(string section, string key, string val, string filePath);
public IniFile(string INIPath)
{
path = INIPath;
}
public void Write(string section, string key, string value)
{
WritePrivateProfileString(section, key, value, path);
}
}
```
使用示例:
```csharp
IniFile ini = new IniFile(@"C:\example.ini");
// 读取值
string value = ini.Read("Section", "Key");
// 写入值
ini.Write("Section", "Key", "Value");
```
相关推荐
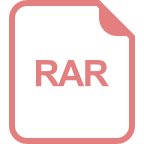
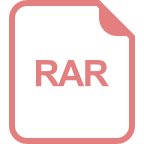
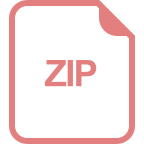








