java excel自动合并行单元格
时间: 2023-09-18 08:08:33 浏览: 71
下面是一个简单的示例代码,演示如何在Java中使用Apache POI库自动合并Excel文件中的行单元格。
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelMergeRows {
public static void main(String[] args) throws IOException {
String inputFilePath = "input.xlsx";
String outputFilePath = "output.xlsx";
String sheetName = "Sheet1";
FileInputStream inputStream = new FileInputStream(new File(inputFilePath));
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheet(sheetName);
Map<Integer, ArrayList<Integer>> mergedRows = new HashMap<>();
// 遍历行
for (int i = 0; i < sheet.getLastRowNum(); i++) {
Row currentRow = sheet.getRow(i);
Row nextRow = sheet.getRow(i + 1);
// 检查当前行和下一行是否需要合并
if (currentRow.getCell(0).getStringCellValue().equals(nextRow.getCell(0).getStringCellValue())) {
// 将当前行和下一行的单元格合并
for (int j = 0; j < currentRow.getLastCellNum(); j++) {
Cell currentCell = currentRow.getCell(j);
Cell nextCell = nextRow.getCell(j);
if (currentCell.getCellType() == Cell.CELL_TYPE_BLANK && nextCell.getCellType() != Cell.CELL_TYPE_BLANK) {
currentCell.setCellValue(nextCell.getStringCellValue());
} else if (nextCell.getCellType() != Cell.CELL_TYPE_BLANK) {
CellStyle currentCellStyle = currentCell.getCellStyle();
CellStyle nextCellStyle = nextCell.getCellStyle();
if (!currentCellStyle.equals(nextCellStyle)) {
currentCell.setCellStyle(nextCellStyle);
}
currentCell.setCellValue(currentCell.getStringCellValue() + "\n" + nextCell.getStringCellValue());
}
}
// 标记需要合并的行
if (!mergedRows.containsKey(i)) {
mergedRows.put(i, new ArrayList<>());
}
mergedRows.get(i).add(i + 1);
// 跳过下一行,因为它已经被合并了
i++;
}
}
// 合并标记的行
for (Map.Entry<Integer, ArrayList<Integer>> entry : mergedRows.entrySet()) {
int rowIndex = entry.getKey();
ArrayList<Integer> mergedIndexes = entry.getValue();
sheet.addMergedRegion(new CellRangeAddress(rowIndex, rowIndex + mergedIndexes.size(), 0, 0));
}
// 保存结果到输出文件
FileOutputStream outputStream = new FileOutputStream(outputFilePath);
workbook.write(outputStream);
workbook.close();
inputStream.close();
outputStream.close();
}
}
```
这个示例代码假设输入文件中的数据存储在名为“Sheet1”的工作表中,并且要将具有相同值的第一列单元格合并为一个单元格。它使用了Apache POI库来处理Excel文件,并使用Map来跟踪需要合并的行。最后,它使用addMergedRegion方法来将标记的行合并为单个单元格,并将结果保存到输出文件中。
阅读全文
相关推荐
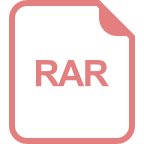





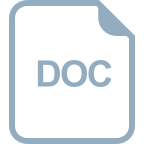







