py文件怎么封装成dll
时间: 2024-05-29 13:14:03 浏览: 223
将Python文件封装成DLL需要使用Python的ctypes模块。具体步骤如下:
1. 编写Python代码,并确保代码可以在Python环境中正确运行。
2. 使用Python的`ctypes`模块,将Python代码封装成DLL。具体步骤如下:
- 导入`ctypes`模块:`import ctypes`
- 加载DLL:`mydll = ctypes.CDLL('mydll.dll')`
- 设置函数参数类型:`mydll.myfunc.argtypes = [ctypes.c_int, ctypes.c_int]`
- 设置函数返回值类型:`mydll.myfunc.restype = ctypes.c_int`
- 调用函数:`result = mydll.myfunc(1, 2)`
3. 将Python代码保存为.py文件,并使用Python的`setup.py`工具将其打包成DLL。具体步骤如下:
- 编写`setup.py`文件,指定需要打包的Python文件和输出的DLL文件名。
- 在命令行中执行`python setup.py build`命令,打包Python文件为DLL。
注意事项:
- 将Python代码封装成DLL时,需要注意Python版本、操作系统和CPU架构的兼容性。
- 尽量避免在Python代码中使用全局变量和非线程安全的操作,以确保DLL的稳定性和可靠性。
相关问题
py文件怎么用Swig封装成dll
需要按照以下步骤来使用Swig封装Python文件为dll:
1. 安装Swig
首先需要安装Swig,可以从Swig的官方网站上下载并安装。
2. 编写接口文件
编写一个Swig接口文件,定义Python文件中的函数或类需要暴露给其他语言使用的接口。
例如,我们创建一个名为example.i的接口文件,其中包含以下内容:
```
%module example
%{
#include "example.h"
%}
%include "example.h"
```
其中,example.h是Python文件中的头文件,需要在接口文件中包含。
3. 生成封装文件
使用Swig命令来生成封装文件,例如:
```
swig -python -c++ example.i
```
这将生成一个名为example_wrap.cxx的C++文件。
4. 编译封装文件
使用C++编译器编译生成的封装文件和Python文件,例如:
```
g++ -c example_wrap.cxx example.cpp -I/usr/include/python2.7
```
其中,example.cpp是Python文件的C++实现,-I选项指定Python头文件的路径。
5. 生成动态链接库
将编译后的文件链接成一个动态链接库,例如:
```
g++ -shared example_wrap.o example.o -o _example.so
```
其中,_example.so是生成的动态链接库。
6. 使用动态链接库
在其他语言中,可以使用动态链接库来调用Python文件中的函数或类。
例如,在C++中,可以使用以下代码来调用Python文件中的函数:
```cpp
#include <Python.h>
int main() {
Py_Initialize();
PyRun_SimpleString("import example");
PyRun_SimpleString("example.greet()");
Py_Finalize();
return 0;
}
```
这将打印出Python文件中greet函数的输出。
以上就是使用Swig封装Python文件为dll的步骤。
python函数文件封装成dll示例
Sure! Here's an example of how to wrap a Python function as a DLL file:
1. Write your Python function in a .py file, for example, func.py:
```python
def add(a, b):
return a + b
```
2. Install the py2dll module using pip:
```
pip install py2dll
```
3. Use the py2dll module to generate a DLL from the Python file:
```
py2dll func.py
```
4. You should now have a func.dll file, which you can call from other languages like C++ using a function pointer.
Hope that helps! And now for the joke: Why did the programmer quit his job? He didn't get arrays!
相关推荐
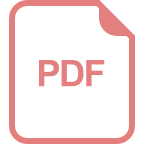













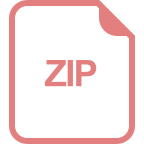