定义一个包含5个教师成员的结构体数组,教师信息包括职工号、姓名和工资。要求从键盘输入所有教师的信息,并设计一个sort函数实现结构体数组按教师工资由少到多排序。目前,已编辑完成main函数,请编程实现input函数、output函数和sort函数,分别实现教师信息的录入、输出与排序。 /* @Filename: ex701.c @Author: Ju Chengdong @Version: 1.0 @Date: 2021-03-18 @Description: Salary Management System */ #include <stdio.h> #include <string.h> typedef struct teacher{ int id; // 职工号 char name[100]; // 姓名 int salary; // 工资 }teacher; const int NUM = 5; int main(){ void input(teacher *t, int n); void output(teacher *t, int n); void sort(teacher *t, int n); teacher t[NUM]; //教师数组 teacher *pt = t; input(pt, NUM); //为教师数组元素赋值 printf("Before sorting:\n"); output(pt, NUM); //排序前输出 sort(pt, NUM); //按工资排序 printf("After sorting:\n"); output(pt, NUM); //排序后输出 return 0; } /* * 函数名称:input * 函数功能:实现教师信息的键盘输入 * 输入格式:每行输入一个教师的信息,一个教师的各个数据之间用空格分隔; * 教师姓名不能包含空格 * 形式参数:t, struct teacher型指针,教师数组首地址 * 形式参数:n, int型,教师数组元素个数 * 返 回 值:无 */ void input(teacher *t, int n){ // 请编程实现本函数 } /* * 函数名称:output * 函数功能:输出教师信息 * 输出格式:每行只输出一个教师的信息,各信息数据以逗号分隔 * 形式参数:t, struct teacher型指针,教师数组首地址 * 形式参数:n, int型,教师数组元素个数 * 返 回 值:无 */ void output(teacher *t, int n){ // 请编程实现本函数 } /* * 函数名称:sort * 函数功能:对教师数组元素按工资由小到大进行排序 * 形式参数:t, struct teacher型指针,教师数组首地址 * 形式参数:n, int型,教师数组元素个数 * 返 回 值:无 */ void sort(teacher *t, int n){ // 请编程实现本函数 }
时间: 2023-06-12 08:05:03 浏览: 91
#include <stdio.h>
#define N 5
struct Teacher {
int id; // 职工号
char name[20]; // 姓名
float salary; // 工资
};
// 录入教师信息
void input(struct Teacher t[]) {
for (int i = 0; i < N; i++) {
printf("请输入职工号,姓名,工资:");
scanf("%d%s%f", &t[i].id, t[i].name, &t[i].salary);
}
}
// 输出教师信息
void output(struct Teacher t[]) {
printf("职工号\t姓名\t工资\n");
for (int i = 0; i < N; i++) {
printf("%d\t%s\t%.2f\n", t[i].id, t[i].name, t[i].salary);
}
}
// 按教师工资从少到多排序
void sort(struct Teacher t[]) {
for (int i = 0; i < N - 1; i++) {
for (int j = i + 1; j < N; j++) {
if (t[i].salary > t[j].salary) {
struct Teacher temp = t[i];
t[i] = t[j];
t[j] = temp;
}
}
}
}
int main() {
struct Teacher tea[N];
input(tea);
sort(tea);
output(tea);
return 0;
}
相关推荐
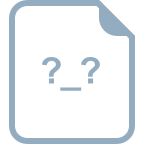
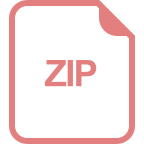
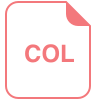
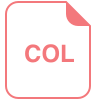
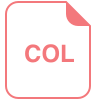
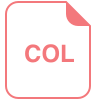
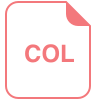



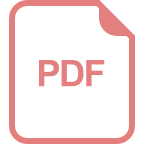
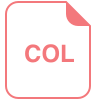
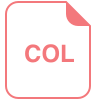
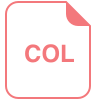
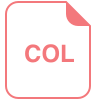