如何保存JS传到后端的数据、以及回显?
时间: 2024-12-08 16:14:50 浏览: 10
在JavaScript中将数据发送到后端通常有几种常见的做法,这里以HTTP请求为例:
1. **Ajax请求**(如使用`fetch()`或jQuery的`$.ajax()`):
- 将数据序列化(如JSON.stringify())成字符串,然后作为POST或PUT请求的body内容。
```javascript
const data = { key: 'value' };
fetch('/api/data', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data),
})
.then(response => response.json())
.then(dataFromServer => console.log('Received:', dataFromServer));
```
2. **Fetch API with FormData**:
对于复杂类型的数据(如文件上传),可能需要使用FormData。
```javascript
const formData = new FormData();
formData.append('key', 'value');
fetch('/api/data', {
method: 'POST',
body: formData,
})
.then(response => response.text());
```
对于数据回显,后端返回响应后,前端通常会解析返回的数据并在合适的视图层展示:
1. **渲染页面**:如果后端返回的是HTML片段,可以替换特定的DOM元素。
```javascript
response.then(html => document.getElementById('display').innerHTML = html);
```
2. **绑定数据到组件**:如果是React或Vue这样的框架,可以用数据绑定技术(如React的`useState`或Vue的`data`)显示数据。
```jsx
function MyComponent() {
const receivedData = useSelector(state => state.receivedData); //假设来自Redux
return <div>{receivedData}</div>;
}
```
阅读全文
相关推荐
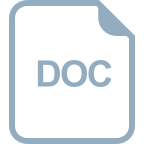
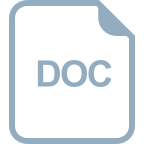
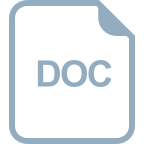

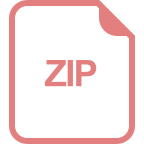
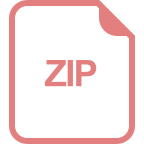
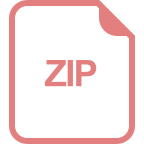
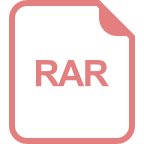
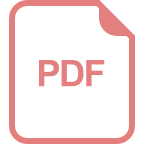
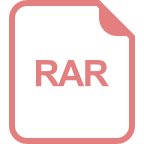
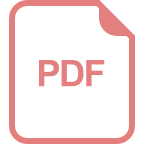
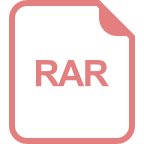
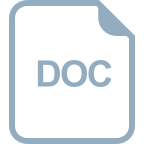
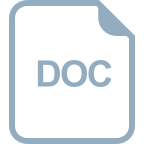
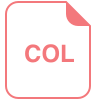



