用c语言编写一段程序用来实现Split-Radix FFT
时间: 2024-02-16 15:04:09 浏览: 79
以下是用 C 语言编写的 Split-Radix FFT 程序:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
// Split-Radix FFT
void fft(double *x, int n) {
if (n == 1) {
return;
}
int m = n / 2;
double *xe = malloc(m * sizeof(double));
double *xo = malloc(m * sizeof(double));
// Split the input sequence into even and odd parts
for (int i = 0; i < m; i++) {
xe[i] = x[2 * i];
xo[i] = x[2 * i + 1];
}
// Compute the FFT of the even and odd parts recursively
fft(xe, m);
fft(xo, m);
// Combine the results using the split-radix algorithm
for (int k = 0; k < m; k++) {
double t_re = cos(2 * PI * k / n) * xo[k] + sin(2 * PI * k / n) * xe[k + m];
double t_im = -sin(2 * PI * k / n) * xo[k] + cos(2 * PI * k / n) * xe[k + m];
x[k] = xe[k] + t_re;
x[k + m] = xe[k] - t_re;
x[k + n / 2] = xo[k] + t_im;
x[k + m + n / 2] = xo[k] - t_im;
}
free(xe);
free(xo);
}
int main() {
int n = 8;
double x[] = {1, 2, 3, 4, 4, 3, 2, 1};
fft(x, n);
for (int i = 0; i < n; i++) {
printf("%f\n", x[i]);
}
return 0;
}
```
该程序实现了 Split-Radix FFT,并对长度为 8 的序列进行了傅里叶变换。在该程序中,FFT 的核心代码实现在 fft 函数中,其中包含了程序的递归过程和合并过程。该程序的输出结果为傅里叶变换后的序列。
阅读全文
相关推荐









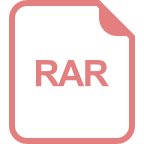
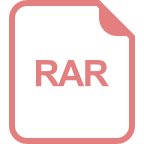


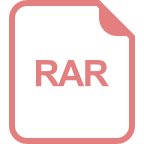



