用c语言写一个简单的modbus tcp主站程序
时间: 2024-05-14 10:07:53 浏览: 14
好的,可以的。下面是一个示例代码:
```c
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<errno.h>
#include<unistd.h>
#include<sys/socket.h>
#include<arpa/inet.h>
#define SERVER_IP "127.0.0.1"
#define SERVER_PORT 502
#define BUFFER_SIZE 1024
// Modbus TCP message header
typedef struct {
uint16_t trans_id; // Transaction ID
uint16_t prot_id; // Protocol ID (0x0000 for Modbus protocol)
uint16_t length; // Length of the message data following the header
uint8_t device; // Device ID (0xFF for Ethernet communications)
uint8_t func_code; // Function code (0x01 for Read Coils function)
uint16_t addr; // Starting address of the coils to be read
uint16_t qty; // Quantity of coiles to be read
} mbtcp_header;
int main()
{
int sockfd = 0;
char buffer[BUFFER_SIZE];
struct sockaddr_in serv_addr;
mbtcp_header mbtcp;
// Create a socket for the Modbus TCP connection
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
printf("Error: could not create socket. %s\n", strerror(errno));
return -1;
}
// Specify the server address and port for the Modbus TCP connection
memset(&serv_addr, 0, sizeof(serv_addr));
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = inet_addr(SERVER_IP);
serv_addr.sin_port = htons(SERVER_PORT);
// Connect to the Modbus TCP server
if (connect(sockfd, (struct sockaddr*)&serv_addr, sizeof(serv_addr)) < 0) {
printf("Error: could not connect to server. %s\n", strerror(errno));
return -1;
}
// Prepare a Modbus TCP message to read coils
mbtcp.trans_id = rand() % 0xFFFF; // Random transaction ID
mbtcp.prot_id = 0x0000; // Modbus protocol ID
mbtcp.length = 0x0006; // Message data length
mbtcp.device = 0xFF; // Ethernet device ID
mbtcp.func_code = 0x01; // Read coils function code
mbtcp.addr = htons(0x0000); // Starting address of the coils to be read
mbtcp.qty = htons(0x0008); // Quantity of coils to be read
// Send the Modbus TCP message
if (send(sockfd, &mbtcp, sizeof(mbtcp), 0) < 0) {
printf("Error: could not send message. %s\n", strerror(errno));
return -1;
}
// Receive the Modbus TCP response
if (recv(sockfd, buffer, BUFFER_SIZE, 0) < 0) {
printf("Error: could not receive response. %s\n", strerror(errno));
return -1;
}
// Close the Modbus TCP connection
close(sockfd);
return 0;
}
```
这是一个简单的示例程序,用于向 Modbus TCP 主站发送一个读取线圈的命令,然后接收 Modbus TCP 从站的响应。在实际使用中,还需要对命令和响应的格式进行具体的定制和解析。
相关推荐
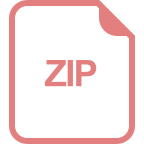














