SpringBoot测试控制器时,什么情况下应该使用MockMvc,什么情况下使用RestTemplate,举例说明
时间: 2024-04-19 19:23:44 浏览: 8
在Spring Boot中测试控制器时,使用MockMvc和RestTemplate的情况取决于你的测试目标和需求。
1. 使用MockMvc:
- 当你只需测试控制器的逻辑,而不需要启动整个应用程序或涉及其他组件时,应该使用MockMvc。它提供了一个模拟的MVC环境,可以发送HTTP请求并验证控制器的行为。
- 适用于单元测试,专注于测试控制器的请求和响应逻辑。
举个例子:
```java
@WebMvcTest(UserController.class)
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetUserById() throws Exception {
mockMvc.perform(get("/users/{id}", 1))
.andExpect(status().isOk())
.andExpect(jsonPath("$.id").value(1))
.andExpect(jsonPath("$.name").value("John Doe"))
.andExpect(jsonPath("$.email").value("johndoe@example.com"));
}
}
```
在上面的例子中,我们使用MockMvc来测试UserController的getUserById方法。通过发送模拟的GET请求并验证响应结果,我们可以测试控制器的行为。
2. 使用RestTemplate:
- 当你需要进行集成测试,涉及到与其他服务进行交互时,应该使用RestTemplate。它是Spring提供的用于发送HTTP请求的客户端工具。
- 适用于对整个应用程序进行端到端测试,包括与其他服务的交互。
举个例子:
```java
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class UserControllerIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testGetUserById() {
ResponseEntity<User> response = restTemplate.getForEntity("/users/{id}", User.class, 1);
User user = response.getBody();
assertEquals(HttpStatus.OK, response.getStatusCode());
assertNotNull(user);
assertEquals(1, user.getId());
assertEquals("John Doe", user.getName());
assertEquals("johndoe@example.com", user.getEmail());
}
}
```
在上面的例子中,我们使用RestTemplate来进行集成测试。通过发送GET请求并解析响应结果,我们可以测试UserController的getUserById方法,并与其他服务进行交互。
总之,使用MockMvc适用于单元测试控制器的逻辑,而使用RestTemplate适用于集成测试,涉及到与其他服务的交互。根据你的测试目标和需求选择合适的工具。
相关推荐
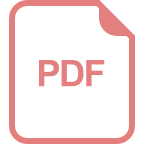
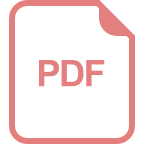
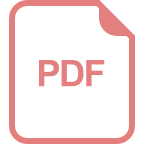













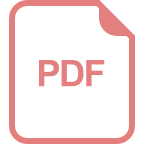
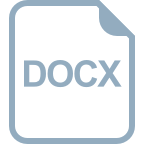