C++使用jsoncpp读取json
时间: 2023-08-16 11:13:22 浏览: 280
要在C++中使用JsonCpp库来读取JSON文件,你可以按照以下步骤进行操作:
1. 首先,确保已经下载并安装了JsonCpp库,并将其包含在你的项目中。
2. 创建一个Json::Value对象来存储解析后的JSON数据。
3. 使用Json::Reader对象来读取JSON文件并解析为Json::Value对象。
下面是一个简单的示例代码,演示了如何使用JsonCpp读取JSON文件:
```cpp
#include <iostream>
#include <fstream>
#include <json/json.h>
int main() {
// 读取 JSON 文件
std::ifstream jsonFile("example.json");
if (!jsonFile.is_open()) {
std::cout << "Failed to open JSON file." << std::endl;
return 1;
}
// 解析 JSON 数据
Json::Value root;
Json::Reader reader;
if (!reader.parse(jsonFile, root)) {
std::cout << "Failed to parse JSON data." << std::endl;
return 1;
}
// 读取 JSON 数据并输出
std::string name = root["name"].asString();
int age = root["age"].asInt();
std::string city = root["city"].asString();
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
std::cout << "City: " << city << std::endl;
return 0;
}
```
在上面的示例中,我们首先打开了一个名为`example.json`的JSON文件,然后使用JsonCpp的Json::Reader对象解析JSON数据,并将其存储在名为`root`的Json::Value对象中。接下来,我们通过访问`root`对象的键值对来获取JSON数据,并将其输出到控制台上。
请确保将示例代码中的`example.json`替换为你实际使用的JSON文件的路径。
这里提供一个CSDN上的相关教程供参考:[https://blog.csdn.net/qq_41453285/article/details/105808014](https://blog.csdn.net/qq_41453285/article/details/105808014)
阅读全文
相关推荐
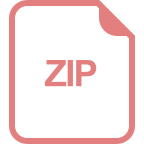
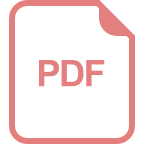
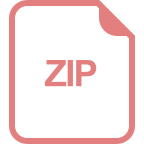

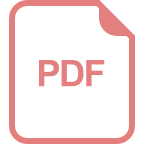
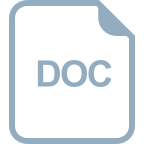

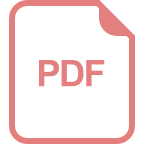
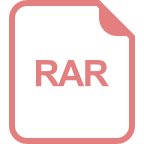
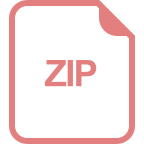







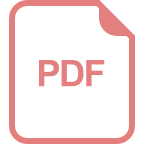