从文件中读取多个学生学号和姓名信息并输出 c++
时间: 2024-09-22 07:01:39 浏览: 19
在C++中,你可以使用`fstream`库来操作文件,包括读取和写入数据。假设你有一个包含学生学号和姓名的数据文件,每行包含两个值(例如以逗号分隔),可以按照下面的方式来读取并输出:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <sstream>
struct Student {
std::string id;
std::string name;
};
int main() {
std::ifstream inputFile("students.txt"); // 打开文件
if (!inputFile) {
std::cerr << "Error opening file." << std::endl;
return 1;
}
std::vector<Student> students; // 存储学生的容器
std::string line;
while (std::getline(inputFile, line)) { // 逐行读取
std::istringstream iss(line);
Student student;
if (!(iss >> student.id >> student.name)) { // 解析一行
std::cerr << "Invalid data format." << std::endl;
continue;
}
students.push_back(student); // 将学生添加到集合中
}
inputFile.close(); // 关闭文件
for (const auto& student : students) { // 输出所有学生的信息
std::cout << "Student ID: " << student.id << ", Name: " << student.name << std::endl;
}
return 0;
}
```
在这个例子中,我们首先定义了一个`Student`结构体存储学号和姓名。然后遍历文件,将每一行解析成`Student`对象,并存入向量`students`中。最后,循环遍历这个向量,输出每个学生的学号和姓名。
相关推荐
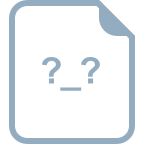
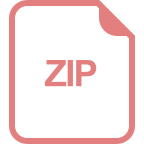
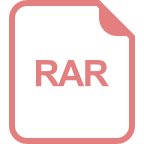














