java jdk1.6 post请求 json对象放body中传递, 接收方从body中获取并输出 demo
时间: 2024-09-20 21:02:56 浏览: 32
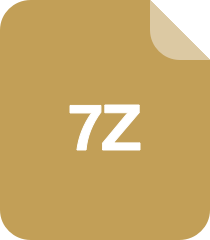
JAVA JDK1.6 API中文帮助文档
在Java JDK 1.6版本中,发送POST请求并携带JSON数据通常使用HTTP客户端库如Apache HttpClient或者更现代的HttpURLConnection。以下是一个简单的示例,展示如何通过HttpClient发送POST请求,将JSON对象作为Body内容:
```java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import java.io.IOException;
public class PostJsonExample {
private static final String JSON_OBJECT = "{\"key\":\"value\"}";
public void sendPostRequest() throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
try {
HttpPost httpPost = new HttpPost("http://example.com/api endpoint"); // 替换为你实际的API地址
// 将JSON转换为StringEntity
HttpEntity entity = new StringEntity(JSON_OBJECT, "application/json");
httpPost.setEntity(entity);
// 发送请求
CloseableHttpResponse response = httpClient.execute(httpPost);
// 检查响应状态码
int responseCode = response.getStatusLine().getStatusCode();
if (responseCode == 200) {
System.out.println("Received response:");
// 读取并打印响应体
HttpEntity respEntity = response.getEntity();
if (respEntity != null) {
System.out.println(EntityUtils.toString(respEntity));
} else {
System.out.println("No content received.");
}
} else {
System.err.println("Failed with status code: " + responseCode);
}
} finally {
httpClient.close();
}
}
public static void main(String[] args) {
try {
PostJsonExample example = new PostJsonExample();
example.sendPostRequest();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,`sendPostRequest`方法会向指定URL发送POST请求,并附带JSON对象。接收端(服务器端)需要解析请求体(即body部分),一般可以使用类似Spring框架的`org.springframework.web.bind.annotation.PostMapping`注解处理JSON请求。
阅读全文
相关推荐
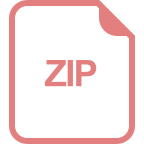
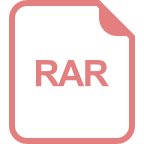
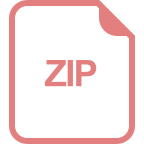
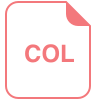
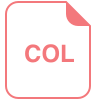
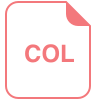
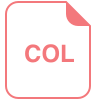
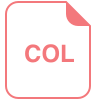

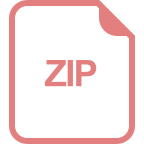
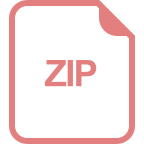
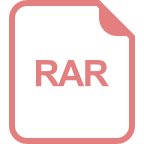
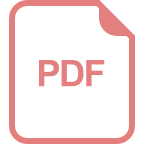
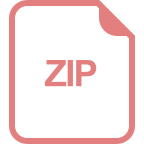