ros2人脸检测代码
时间: 2024-09-19 17:18:05 浏览: 88
ROS 2 (Robot Operating System) 是一个开源的机器人操作系统,它提供了一套完整的框架来支持复杂系统的设计、部署和交互。对于人脸检测,通常会使用计算机视觉库如`cv_bridge`和深度学习框架如`tf2_ros`配合深度学习模型来实现。
在ROS 2中,常用的人脸检测算法有基于深度学习的方法,比如OpenCV中的`dnn`模块,结合预训练的人脸检测模型如MTCNN (Multi-task Cascaded Convolutional Networks) 或者RetinaFace等。以下是一个简单的示例:
```cpp
#include <image_transport/image_transport.h>
#include <sensor_msgs/image_msg.h>
#include <opencv2/opencv.hpp>
#include <opencv2/dnn/dnn.hpp>
class FaceDetectorNode : public rclcpp::Node
{
public:
FaceDetectorNode(rclcpp::node::NodeOptions options)
: rclcpp::Node("face_detector", options),
image_sub_(this->create_subscription<sensor_msgs::msg::Image>("input_image", 10, std::bind(&FaceDetectorNode::image_callback, this, _1))),
output_pub_(this->create_publisher<sensor_msgs::msg::Image>("output_face", 10))
{
}
private:
void image_callback(const sensor_msgs::msg::Image::SharedPtr msg)
{
cv_bridge:: CvBridge bridge;
cv::Mat input_img;
bridge.imgmsg_to_cv2(*msg, input_img);
// Load pre-trained model
dnn::Net net = dnn::readNetFromTensorflow("path/to/your/frozen.pb");
// Resize and preprocess the image
cv::resize(input_img, input_img, cv::Size(), 0.5, 0.5);
cv::Mat blob = dnn.blobFromImage(input_img, 1.0, cv::Size(300, 300), {104, 177, 123}, false, false);
// Forward pass through the network
net.setInput(blob);
cv::Mat detections = net.forward();
// Draw bounding boxes around detected faces
for (int i = 0; i < detections.size[2]; ++i)
{
float confidence = detections.at<float>(0, i, 2);
if (confidence > threshold)
{
int left = static_cast<int>(detections.at<float>(0, i, 3) * input_img.cols);
int top = static_cast<int>(detections.at<float>(0, i, 4) * input_img.rows);
int right = static_cast<int>((detections.at<float>(0, i, 3) + detections.at<float>(0, i, 5)) * input_img.cols);
int bottom = static_cast<int>((detections.at<float>(0, i, 4) + detections.at<float>(0, i, 6)) * input_img.rows);
cv::rectangle(input_img, cv::Point(left, top), cv::Point(right, bottom), cv::Scalar(0, 255, 0), 2);
}
}
// Publish the processed image
sensor_msgs::msg::Image out_msg;
bridge.cv2ToImgmsg(input_img, "bgr8", &out_msg);
output_pub_.publish(out_msg);
}
rclcpp::Subscription<sensor_msgs::msg::Image>::SharedPtr image_sub_;
rclcpp::Publisher<sensor_msgs::msg::Image>::SharedPtr output_pub_;
double threshold; // You can set a detection confidence threshold here
};
int main(int argc, char ** argv)
{
rclcpp::init(argc, argv);
auto node = FaceDetectorNode::make_shared();
rclcpp::spin(node);
return 0;
}
```
注意,这个示例假设你已经将一个人脸检测模型(如TensorFlow frozen graph文件)转换成了OpenCV可以加载的格式,并设置了合适的阈值。实际使用时,你需要根据你的需求调整模型路径和参数。
阅读全文
相关推荐
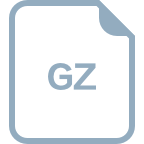
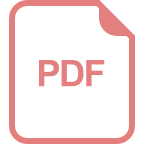

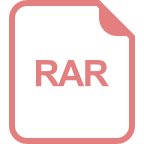
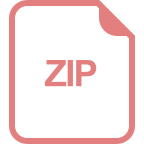
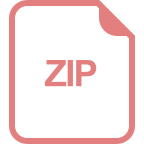
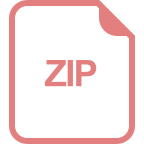
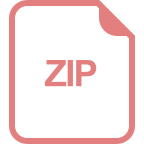
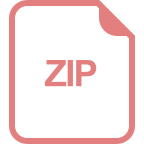
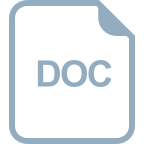
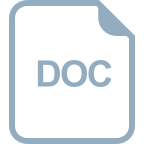
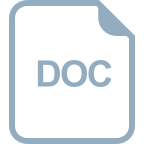
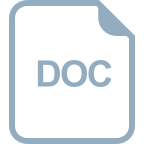
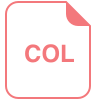


