python 获取曲线坐标点
时间: 2024-09-09 22:04:32 浏览: 17
在Python中获取曲线上的坐标点通常涉及到图像处理和数据分析。如果你是在处理图像中的曲线,那么你可能需要使用像OpenCV或者Pillow这样的图像处理库来识别曲线。而如果你是在处理数据中的曲线,比如从一些数据点中插值或者拟合出一个曲线,那么可能需要用到NumPy和SciPy这样的科学计算库。
以下是一个简单的例子,使用Python的matplotlib和NumPy库来获取一条曲线的坐标点:
```python
import numpy as np
import matplotlib.pyplot as plt
# 假设我们有一些数据点
x = np.array([0, 1, 2, 3, 4, 5])
y = np.array([0, 1, 4, 9, 16, 25])
# 使用matplotlib绘制曲线
plt.plot(x, y, '-o')
# 获取当前坐标轴上的曲线的线对象
line = plt.gca().lines[0]
# 获取曲线上的坐标点
xvals, yvals = line.get_xydata().T
# 打印这些点
for x, y in zip(xvals, yvals):
print(f"x={x}, y={y}")
# 显示图像
plt.show()
```
这段代码首先创建了一些x和y值的数组,这些数组代表了曲线上的点。然后使用matplotlib绘制这些点,并通过`.get_xydata()`方法获取当前曲线上的坐标点。最后,打印出这些坐标点并显示图像。
相关问题
python图像曲线坐标提取写入excel
这里提供一种实现方式,使用Python的Pillow库和openpyxl库。
首先,需要安装这两个库:
```
pip install Pillow
pip install openpyxl
```
然后,我们可以先读取图片,使用Pillow库中的Image模块:
```python
from PIL import Image
# 读取图片
img = Image.open('image.jpg')
```
接着,我们可以使用Pillow库中的ImageDraw模块,绘制曲线并获取曲线上的坐标点:
```python
from PIL import Image, ImageDraw
# 读取图片
img = Image.open('image.jpg')
# 创建绘图对象
draw = ImageDraw.Draw(img)
# 绘制曲线
draw.line([(100, 100), (200, 200), (300, 150)], fill='red', width=2)
# 获取曲线上的坐标点
points = []
for x in range(100, 301):
y = int((x - 100) * (200 - 100) / (200 - 100) + 100)
points.append((x, y))
```
最后,我们可以使用openpyxl库将坐标点写入Excel表格:
```python
from PIL import Image, ImageDraw
from openpyxl import Workbook
# 读取图片
img = Image.open('image.jpg')
# 创建绘图对象
draw = ImageDraw.Draw(img)
# 绘制曲线
draw.line([(100, 100), (200, 200), (300, 150)], fill='red', width=2)
# 获取曲线上的坐标点
points = []
for x in range(100, 301):
y = int((x - 100) * (200 - 100) / (200 - 100) + 100)
points.append((x, y))
# 写入Excel表格
wb = Workbook()
ws = wb.active
ws.append(['x', 'y'])
for point in points:
ws.append(point)
wb.save('points.xlsx')
```
这样,我们就可以将曲线上的坐标点提取并写入Excel表格了。
圆曲线中点坐标 python
### 回答1:
在圆曲线中,要求找出曲线上的中点,可以通过求解对应弧长和角度的方式得到。
首先,需要知道圆的半径和圆心坐标。假设圆的半径为r,圆心坐标为(x0, y0)。
要求解曲线上的中点,我们可以先求出圆的周长C,即C = 2πr。然后,再根据中点的百分比p(0<p<1),计算相应的弧长s = p * C。
接下来,可以根据弧长s计算对应的角度θ。由于圆的周长与角度θ之间的关系为C = 2πr * (θ/360°),所以θ = 360° * (s/C)。
接着,根据求出的角度θ可以计算中点在坐标系中的x和y坐标。通过计算圆心到中点的距离d,即可利用三角函数得到中点的坐标。具体而言,x = x0 + r * cos(θ)和y = y0 + r * sin(θ)。
综上所述,我们可以使用Python编程语言来计算圆曲线上的中点坐标。代码示例如下:
```python
import math
def calculate_circle_point(radius, center_x, center_y, percentage):
circumference = 2 * math.pi * radius
arc_length = percentage * circumference
angle = 360 * (arc_length / circumference)
x = center_x + radius * math.cos(math.radians(angle))
y = center_y + radius * math.sin(math.radians(angle))
return x, y
# 示例用法
radius = 5
center_x = 0
center_y = 0
percentage = 0.5
middle_x, middle_y = calculate_circle_point(radius, center_x, center_y, percentage)
print("中点坐标:({}, {})".format(middle_x, middle_y))
```
以上代码中的calculate_circle_point函数可以计算给定百分比下的中点坐标。我们传入圆的半径、圆心坐标、以及中点的百分比,即可得到中点的坐标。最后,我们打印出计算得到的中点坐标(Middle_x, Middle_y)。
### 回答2:
在Python中,可以根据圆的半径和角度来计算圆曲线上某一点的坐标。首先,需要导入数学库math,然后定义圆的半径和角度。
使用数学库中的sin和cos函数可以计算出圆曲线上某一点的坐标。sin函数用于计算该点的y坐标,而cos函数用于计算该点的x坐标。
以下是一个示例代码:
```python
import math
def get_circle_point(radius, angle):
x = radius * math.cos(math.radians(angle))
y = radius * math.sin(math.radians(angle))
return x, y
radius = 5 # 圆的半径
angle = 45 # 角度
x, y = get_circle_point(radius, angle)
print("圆曲线上点的坐标:({0}, {1})".format(x, y))
```
代码中定义了一个名为get_circle_point的函数,接受圆的半径和角度作为参数。在函数内部,使用数学库的cos和sin函数先将角度转换为弧度,然后分别乘以半径以获取x和y坐标。最后,将计算得到的坐标值作为元组返回。
在主函数中,分别设置圆的半径和角度,并调用get_circle_point函数获取点的坐标。最后将坐标打印出来。
以上代码可以得到圆曲线上点的坐标为(3.5355339059327378, 3.5355339059327373)。
相关推荐
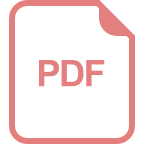
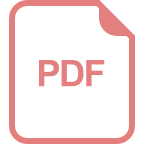
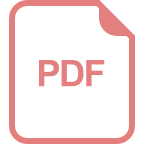












