springboot sop wsdl服务端实现,可接收复杂参数,并使用postman发起请求
时间: 2024-04-12 21:26:39 浏览: 214
您好!您可以使用Spring Boot来实现SOAP(Simple Object Access Protocol)WS(Web Services)的服务端。下面是一个简单的示例代码来演示如何实现:
1. 首先,您需要在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Web Services -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
<!-- Apache CXF -->
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-spring-boot-starter-jaxws</artifactId>
<version>3.4.1</version>
</dependency>
</dependencies>
```
2. 创建一个SOAP服务接口,例如:
```java
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebService;
@WebService
public interface MySoapService {
@WebMethod
String processRequest(@WebParam(name = "param") MyComplexParam param);
}
```
3. 创建一个复杂参数类,例如:
```java
public class MyComplexParam {
private String name;
private int age;
// 其他属性和方法
// Getter和Setter方法省略
}
```
4. 创建一个实现SOAP服务接口的类,例如:
```java
import javax.jws.WebService;
@WebService(endpointInterface = "com.example.MySoapService")
public class MySoapServiceImpl implements MySoapService {
@Override
public String processRequest(MyComplexParam param) {
// 处理请求的逻辑
return "Hello, " + param.getName() + "! Your age is " + param.getAge() + ".";
}
}
```
5. 创建一个配置类,用于发布SOAP服务,例如:
```java
import org.apache.cxf.Bus;
import org.apache.cxf.bus.spring.SpringBus;
import org.apache.cxf.jaxws.EndpointImpl;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import javax.xml.ws.Endpoint;
@Configuration
public class WebServiceConfig {
@Bean
public MySoapService mySoapService() {
return new MySoapServiceImpl();
}
@Bean(name = Bus.DEFAULT_BUS_ID)
@Primary
public SpringBus springBus() {
return new SpringBus();
}
@Bean
public Endpoint endpoint() {
EndpointImpl endpoint = new EndpointImpl(springBus(), mySoapService());
endpoint.publish("/mySoapService"); // 指定发布的URL路径
return endpoint;
}
}
```
6. 运行Spring Boot应用程序,您的SOAP服务将在指定的URL路径上发布。
7. 使用Postman或其他工具来发起SOAP请求。您可以将复杂参数作为XML或JSON进行传输,具体取决于您在请求中使用的Content-Type。
希望这些代码示例能帮助您实现Spring Boot SOAP WS的服务端,并成功接收到复杂参数。如有需要,请根据您的实际情况进行适当的修改。
阅读全文
相关推荐
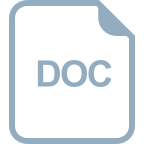
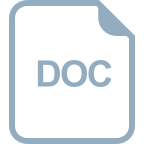
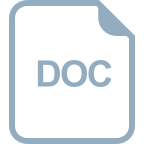
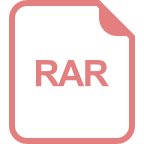
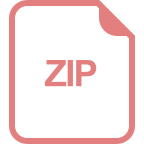
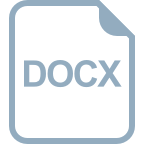
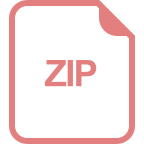
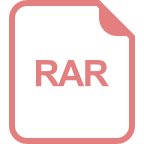
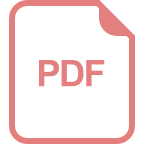
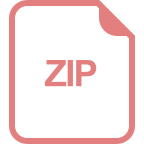
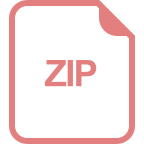
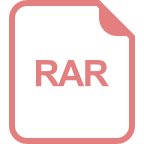
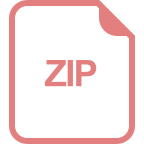
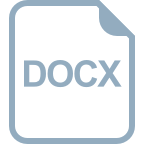
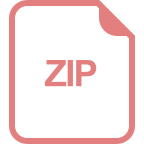
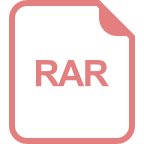
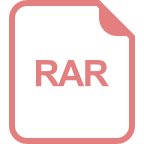