export default class WS
时间: 2024-01-04 15:19:18 浏览: 27
export default class WS 是一个使用了ES6模块化语法的代码片段,它导出了一个名为WS的类作为默认导出。这意味着在其他文件中引入该模块时,可以使用 import WS from 'module' 来获取该类。
范例:
```javascript
// module.js
export default class WS {
constructor() {
// 类的构造函数
}
// 类的方法
method1() {
// 方法实现
}
method2() {
// 方法实现
}
}
// main.js
import WS from 'module';
const ws = new WS();
ws.method1();
ws.method2();
```
在上面的例子中,我们定义了一个名为WS的类,并将其作为默认导出。在main.js中,我们使用import语句将WS类导入,并创建了一个ws实例,然后可以调用ws的方法method1和method2。
相关问题
express-ws+vue3实现微信聊天代码
首先,您需要安装 `express-ws` 和 `vue3`。可以使用以下命令进行安装:
在服务器端(使用 Express.js):
```bash
npm install express express-ws
```
在客户端(使用 Vue.js):
```bash
npm install vue@next
```
接下来,您可以按照以下步骤实现微信聊天的代码:
1. 服务器端代码(Express.js + express-ws):
```javascript
// server.js
const express = require('express');
const expressWs = require('express-ws');
const app = express();
expressWs(app);
const clients = [];
app.ws('/chat', (ws, req) => {
clients.push(ws);
ws.on('message', (msg) => {
// 广播收到的消息给所有客户端
clients.forEach((client) => {
client.send(msg);
});
});
ws.on('close', () => {
// 移除断开连接的客户端
const index = clients.indexOf(ws);
if (index !== -1) {
clients.splice(index, 1);
}
});
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
2. 客户端代码(Vue.js):
```html
<!-- App.vue -->
<template>
<div>
<h1>微信聊天</h1>
<div class="chat-box">
<div v-for="(message, index) in messages" :key="index" class="message">{{ message }}</div>
</div>
<input v-model="inputMessage" @keyup.enter="sendMessage" type="text" placeholder="输入消息" />
</div>
</template>
<script>
import { ref } from 'vue';
export default {
name: 'App',
setup() {
const inputMessage = ref('');
const messages = ref([]);
const ws = new WebSocket('ws://localhost:3000/chat');
ws.onmessage = (event) => {
// 接收到服务器发送的消息
messages.value.push(event.data);
};
const sendMessage = () => {
// 发送消息到服务器
if (inputMessage.value.trim() !== '') {
ws.send(inputMessage.value);
inputMessage.value = '';
}
};
return {
inputMessage,
messages,
sendMessage,
};
},
};
</script>
<style>
.message {
margin-bottom: 10px;
}
</style>
```
3. 在一个新的文件中,创建一个 Vue 实例并将其挂载到页面上:
```javascript
// main.js
import { createApp } from 'vue';
import App from './App.vue';
createApp(App).mount('#app');
```
在完成上述步骤后,您可以运行服务器端代码(`node server.js`),然后在浏览器中访问客户端代码,即可实现微信聊天功能。请确保服务器和客户端代码在同一台机器上运行,并且端口号(3000)没有被占用。
当有客户端发送消息时,服务器将广播该消息给所有连接的客户端,从而实现聊天功能。
ImportError: dynamic module does not define module export function (PyInit_cv_bridge_boost)
在你的错误信息中,ImportError: dynamic module does not define module export function (PyInit_cv_bridge_boost),这可能是由于ROS自带的cv_bridge只支持Python2而不是Python3导致的。为了解决这个问题,你需要编译一个适用于Python3的cv_bridge模块。
以下是解决方案:
1. 首先,确保你在系统的真实环境中操作,而不是在conda创建的虚拟环境中。打开一个新终端,并运行conda deactivate命令,确保退出所有虚拟环境。
2. 进入Python3环境并安装相关依赖包:
```
sudo apt-get install python-catkin-tools python3-dev python3-catkin-pkg-modules python3-numpy python3-yaml ros-melodic-cv-bridge
```
注意,根据你使用的ROS版本可能会有略微不同的依赖包名称。
3. 创建一个工作空间用于存放待编译的cv_bridge文件:
```
mkdir -p catkin_ws_for_cvbridge/src
```
4. 设置参数:
```
cd catkin_ws_for_cvbridge
catkin config -DPYTHON_EXECUTABLE=/usr/bin/python3 -DPYTHON_INCLUDE_DIR=/usr/include/python3.6m -DPYTHON_LIBRARY=/usr/lib/x86_64-linux-gnu/libpython3.6m.so
```
注意,这里的参数根据你系统自带的Python版本进行设置,16.04使用Python3.5,18.04使用Python3.6。
5. 告诉catkin将构建的包安装到指定位置:
```
catkin config --install
```
6. 在catkin_workspace工作空间中克隆cv_bridge:
```
git clone https://github.com/ros-perception/vision_opencv.git src/vision_opencv
```
7. 查看适合的cv_bridge版本:
```
apt-cache show ros-melodic-cv-bridge | grep Version
```
8. 编译cv_bridge:
```
catkin build cv_bridge
```
如果一切顺利,应该能够成功编译cv_bridge模块。
9. 最后,确保你将编译后的cv_bridge包添加到ROS的环境变量中。
通过以上步骤,你应该能够解决ImportError: dynamic module does not define module export function (PyInit_cv_bridge_boost)的问题。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [在Python3环境中使用ROS的cv_bridge](https://download.csdn.net/download/weixin_38703794/14036198)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [【bug】ImportError: dynamic module does not define module export function (PyInit_cv_bridge_boost)](https://blog.csdn.net/qq_36497369/article/details/128853820)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
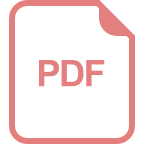
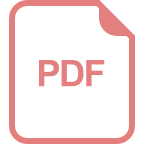
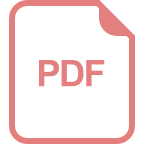












