PHP 的 OpenSSL 扩展中,非对称加密的相关函数有:
时间: 2024-03-11 10:45:59 浏览: 17
PHP 的 OpenSSL 扩展提供了一系列非对称加密的相关函数,用于生成密钥对、加密和解密数据等操作。其中比较常用的函数包括:
1. `openssl_pkey_new`:生成一个新的公私钥对。
2. `openssl_pkey_export`:将私钥导出。
3. `openssl_pkey_get_private`:将 PEM 格式的私钥导入。
4. `openssl_public_encrypt`:使用公钥加密数据。
5. `openssl_private_decrypt`:使用私钥解密数据。
6. `openssl_sign`:使用私钥对数据进行签名。
7. `openssl_verify`:使用公钥验证签名。
这些函数都可以使用 OpenSSL 扩展中提供的默认算法,也可以通过传递参数来指定算法和密钥长度等信息。
相关问题
openssl 对称加密
OpenSSL 是一个开源的加密库,支持对称加密、非对称加密、哈希算法等多种加密方式。对称加密是指加密和解密使用相同的密钥,常见的对称加密算法有 DES、3DES、AES 等。
在 OpenSSL 中,使用 EVP 接口进行对称加密操作。下面是一个使用 AES-256-CBC 算法进行加密和解密的示例代码:
```c
#include <openssl/evp.h>
#include <string.h>
int aes_encrypt(const unsigned char *plaintext, int plaintext_len,
const unsigned char *key, const unsigned char *iv,
unsigned char *ciphertext)
{
EVP_CIPHER_CTX *ctx;
int len;
int ciphertext_len;
/* Create and initialise the context */
if(!(ctx = EVP_CIPHER_CTX_new())) return -1;
/* Initialise the encryption operation. */
if(1 != EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv))
return -1;
/* Provide the message to be encrypted, and obtain the encrypted output.
* EVP_EncryptUpdate can be called multiple times if necessary
*/
if(1 != EVP_EncryptUpdate(ctx, ciphertext, &len, plaintext, plaintext_len))
return -1;
ciphertext_len = len;
/* Finalise the encryption. */
if(1 != EVP_EncryptFinal_ex(ctx, ciphertext + len, &len)) return -1;
ciphertext_len += len;
/* Clean up */
EVP_CIPHER_CTX_free(ctx);
return ciphertext_len;
}
int aes_decrypt(const unsigned char *ciphertext, int ciphertext_len,
const unsigned char *key, const unsigned char *iv,
unsigned char *plaintext)
{
EVP_CIPHER_CTX *ctx;
int len;
int plaintext_len;
int ret;
/* Create and initialise the context */
if(!(ctx = EVP_CIPHER_CTX_new())) return -1;
/* Initialise the decryption operation. */
if(1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv))
return -1;
/* Provide the message to be decrypted, and obtain the plaintext output.
* EVP_DecryptUpdate can be called multiple times if necessary
*/
if(1 != EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, ciphertext_len))
return -1;
plaintext_len = len;
/* Finalise the decryption. */
ret = EVP_DecryptFinal_ex(ctx, plaintext + len, &len);
if(ret > 0) {
plaintext_len += len;
} else {
/* Error */
EVP_CIPHER_CTX_free(ctx);
return -1;
}
/* Clean up */
EVP_CIPHER_CTX_free(ctx);
return plaintext_len;
}
```
其中,`aes_encrypt` 函数用于加密,`aes_decrypt` 函数用于解密。`plaintext` 是明文,`plaintext_len` 是明文长度,`key` 是密钥,`iv` 是初始化向量,`ciphertext` 是密文,`ciphertext_len` 是密文长度,`plaintext` 是解密后的明文。
c++ openssl 使用ECC算法对大文件进行非对称加密和解密示例代码
以下是使用 OpenSSL 库对大文件进行 ECC 算法的非对称加密和解密的示例代码:
```c++
#include <openssl/evp.h>
#include <openssl/ec.h>
#include <openssl/ecdsa.h>
#include <openssl/rand.h>
#include <openssl/pem.h>
#include <iostream>
#include <fstream>
using namespace std;
// 加密函数
int ecc_encrypt(unsigned char* plaintext, int plaintext_len, unsigned char* ciphertext, int* ciphertext_len, EC_KEY* ec_key)
{
EVP_PKEY* pkey = EVP_PKEY_new();
EVP_PKEY_set1_EC_KEY(pkey, ec_key);
EVP_PKEY_CTX* ctx = EVP_PKEY_CTX_new(pkey, nullptr);
if (ctx == nullptr) {
cerr << "Error: EVP_PKEY_CTX_new() failed" << endl;
return -1;
}
if (EVP_PKEY_encrypt_init(ctx) <= 0) {
cerr << "Error: EVP_PKEY_encrypt_init() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
if (EVP_PKEY_CTX_set_rsa_padding(ctx, RSA_PKCS1_OAEP_PADDING) <= 0) {
cerr << "Error: EVP_PKEY_CTX_set_rsa_padding() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
if (EVP_PKEY_CTX_set_rsa_oaep_md(ctx, EVP_sha256()) <= 0) {
cerr << "Error: EVP_PKEY_CTX_set_rsa_oaep_md() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
if (EVP_PKEY_encrypt(ctx, ciphertext, ciphertext_len, plaintext, plaintext_len) <= 0) {
cerr << "Error: EVP_PKEY_encrypt() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
EVP_PKEY_CTX_free(ctx);
EVP_PKEY_free(pkey);
return 0;
}
// 解密函数
int ecc_decrypt(unsigned char* ciphertext, int ciphertext_len, unsigned char* plaintext, int* plaintext_len, EC_KEY* ec_key)
{
EVP_PKEY* pkey = EVP_PKEY_new();
EVP_PKEY_set1_EC_KEY(pkey, ec_key);
EVP_PKEY_CTX* ctx = EVP_PKEY_CTX_new(pkey, nullptr);
if (ctx == nullptr) {
cerr << "Error: EVP_PKEY_CTX_new() failed" << endl;
return -1;
}
if (EVP_PKEY_decrypt_init(ctx) <= 0) {
cerr << "Error: EVP_PKEY_decrypt_init() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
if (EVP_PKEY_CTX_set_rsa_padding(ctx, RSA_PKCS1_OAEP_PADDING) <= 0) {
cerr << "Error: EVP_PKEY_CTX_set_rsa_padding() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
if (EVP_PKEY_CTX_set_rsa_oaep_md(ctx, EVP_sha256()) <= 0) {
cerr << "Error: EVP_PKEY_CTX_set_rsa_oaep_md() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
if (EVP_PKEY_decrypt(ctx, plaintext, plaintext_len, ciphertext, ciphertext_len) <= 0) {
cerr << "Error: EVP_PKEY_decrypt() failed" << endl;
EVP_PKEY_CTX_free(ctx);
return -1;
}
EVP_PKEY_CTX_free(ctx);
EVP_PKEY_free(pkey);
return 0;
}
int main(int argc, char* argv[])
{
// 生成 ECC 密钥对
EC_KEY* ec_key = EC_KEY_new_by_curve_name(NID_secp384r1);
if (ec_key == nullptr) {
cerr << "Error: EC_KEY_new_by_curve_name() failed" << endl;
return -1;
}
if (EC_KEY_generate_key(ec_key) <= 0) {
cerr << "Error: EC_KEY_generate_key() failed" << endl;
EC_KEY_free(ec_key);
return -1;
}
// 打开需要加密的文件
ifstream fin("plaintext.txt", ios::binary);
if (!fin) {
cerr << "Error: cannot open file" << endl;
EC_KEY_free(ec_key);
return -1;
}
// 计算需要加密的文件的长度
fin.seekg(0, ios::end);
int plaintext_len = fin.tellg();
fin.seekg(0, ios::beg);
// 读入需要加密的文件
unsigned char* plaintext = new unsigned char[plaintext_len];
fin.read((char*)plaintext, plaintext_len);
fin.close();
// 计算加密后的文件的长度
int ciphertext_len = EC_GROUP_get_degree(EC_KEY_get0_group(ec_key)) / 8 + 1 + plaintext_len + EVP_MD_size(EVP_sha256());
unsigned char* ciphertext = new unsigned char[ciphertext_len];
// 加密
int ret = ecc_encrypt(plaintext, plaintext_len, ciphertext, &ciphertext_len, ec_key);
if (ret != 0) {
EC_KEY_free(ec_key);
delete[] plaintext;
delete[] ciphertext;
return -1;
}
// 将加密后的文件写入磁盘
ofstream fout("ciphertext.bin", ios::binary);
if (!fout) {
cerr << "Error: cannot open file" << endl;
EC_KEY_free(ec_key);
delete[] plaintext;
delete[] ciphertext;
return -1;
}
fout.write((char*)ciphertext, ciphertext_len);
fout.close();
// 打开需要解密的文件
fin.open("ciphertext.bin", ios::binary);
if (!fin) {
cerr << "Error: cannot open file" << endl;
EC_KEY_free(ec_key);
delete[] plaintext;
delete[] ciphertext;
return -1;
}
// 计算需要解密的文件的长度
fin.seekg(0, ios::end);
ciphertext_len = fin.tellg();
fin.seekg(0, ios::beg);
// 读入需要解密的文件
ciphertext = new unsigned char[ciphertext_len];
fin.read((char*)ciphertext, ciphertext_len);
fin.close();
// 计算解密后的文件的长度
plaintext_len = ciphertext_len - EC_GROUP_get_degree(EC_KEY_get0_group(ec_key)) / 8 - 1 - EVP_MD_size(EVP_sha256());
plaintext = new unsigned char[plaintext_len];
// 解密
ret = ecc_decrypt(ciphertext, ciphertext_len, plaintext, &plaintext_len, ec_key);
if (ret != 0) {
EC_KEY_free(ec_key);
delete[] plaintext;
delete[] ciphertext;
return -1;
}
// 将解密后的文件写入磁盘
fout.open("decrypted.txt", ios::binary);
if (!fout) {
cerr << "Error: cannot open file" << endl;
EC_KEY_free(ec_key);
delete[] plaintext;
delete[] ciphertext;
return -1;
}
fout.write((char*)plaintext, plaintext_len);
fout.close();
EC_KEY_free(ec_key);
delete[] plaintext;
delete[] ciphertext;
return 0;
}
```
这个示例代码使用了 OpenSSL 库提供的 EVP 接口进行 ECC 算法的加密和解密。其中,`ecc_encrypt` 函数使用 ECC 密钥对对明文进行加密,`ecc_decrypt` 函数使用 ECC 密钥对对密文进行解密。在主函数中,首先生成一个 ECC 密钥对,然后读入需要加密的文件,计算加密后的文件的长度,进行加密,并将加密后的文件写入磁盘。接着,读入需要解密的文件,计算解密后的文件的长度,进行解密,并将解密后的文件写入磁盘。注意,在实际应用中,需要保证密文的安全性,可以使用对称加密算法对密文进行进一步的保护。
相关推荐
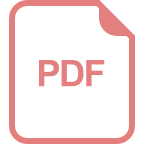
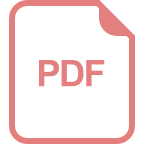
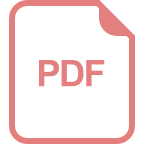












