切换到正确的frame
时间: 2024-04-17 19:26:04 浏览: 35
要切换到正确的 frame,可以使用 `driver.switch_to.frame()` 方法。您需要提供 frame 的定位方式和值作为参数。
以下是一个示例,假设您要切换到 ID 为 "frameId" 的 frame:
```python
frame = driver.find_element(By.ID, "frameId")
driver.switch_to.frame(frame)
```
如果 frame 是通过 name 或索引进行定位的,可以使用以下方式:
通过 name 定位:
```python
driver.switch_to.frame("frameName")
```
通过索引定位:
```python
driver.switch_to.frame(0) # 切换到第一个 frame
```
请根据您的实际情况,将示例代码中的 frame 定位方式和值替换为正确的值。切换到正确的 frame 后,您就可以在该 frame 内执行其他操作了。
相关问题
def switch_to_iframe(self, locator): # 等待iframe加载完成并切换到iframe locator = self.made_locator(locator) iframe = self.driver.find_element(locator) self.driver.switch_to.frame(iframe) WebDriverWait(self.driver, 10).until(EC.frame_to_be_available_and_switch_to_it(locator))
您的代码看起来几乎正确,但有一点需要注意。在您的代码中,您先找到了iframe元素,然后再使用`switch_to.frame()`方法切换到该iframe。然而,根据我理解的需求,您应该先等待iframe加载完成并切换到iframe,然后再找到iframe元素。
以下是修正后的代码:
```python
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
def switch_to_iframe(self, locator):
# 等待iframe加载完成并切换到iframe
locator = self.made_locator(locator)
WebDriverWait(self.driver, 10).until(EC.frame_to_be_available_and_switch_to_it(locator))
```
在这个修正后的代码中,我们首先使用`made_locator()`方法来处理传入的定位器参数,并将其赋值给`locator`。
然后,我们使用`WebDriverWait`和`EC.frame_to_be_available_and_switch_to_it()`来等待iframe加载完成并切换到iframe。这样做可以确保在找到iframe元素之前,WebDriver会等待一段时间。
请注意,我假设您已经在类中定义了`made_locator()`方法来处理定位器参数并返回正确的定位器对象。如果没有,请根据自己的需求进行调整。
希望这次的修正对您有所帮助!如果还有其他问题,请随时提问。
如何在程序运行过程中切换摄像头源(比如从内置摄像头切换到外置摄像头)?
在Python的`cv2.VideoCapture`中,你可以通过修改设备索引来动态切换摄像头源。默认情况下,`VideoCapture(0)`表示内置摄像头,而`VideoCapture(1)`、`VideoCapture(2)`等则对应于可用的其他外置摄像头。
如果你想切换摄像头,可以在需要的时候更改这个参数,例如:
```python
# 切换到内置摄像头
cap = cv2.VideoCapture(0)
# 检查是否成功打开摄像头
if not cap.isOpened():
print("无法打开内置摄像头")
# 想切换到外置摄像头(假设第二台外置摄像头)
cap.release() # 先释放当前摄像头
cap = cv2.VideoCapture(1)
```
这里先关闭当前的摄像头(如果之前是内建的),然后尝试打开设备编号为1的摄像头。记得检查每个`isOpened()`方法返回值,确认摄像头已经成功打开。
请注意,实际可用的摄像头设备索引可能会因电脑配置不同而变化,你可以通过遍历所有可用设备来确定正确的索引:
```python
import cv2
cam_list = [i for i in range(cv2.CAP_PROP_FRAME_COUNT)] # 获取所有摄像设备列表
active_cam = cam_list[0] # 初始化为第一个设备
while True:
try:
cap = cv2.VideoCapture(active_cam)
if cap.isOpened(): # 如果能打开则继续使用
break
except Exception as e:
print(f"无法打开摄像头 {active_cam}: {e}")
active_cam += 1 # 尝试下一个设备
cap.release()
cap = cv2.VideoCapture(active_cam) # 最终选择的设备打开
```
这样在程序运行过程中就可以动态地切换到指定的摄像头了。
阅读全文
相关推荐
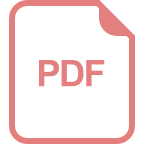
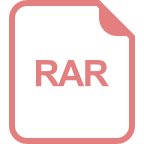
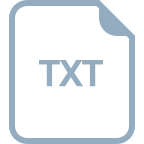
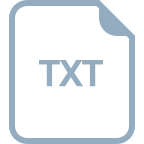
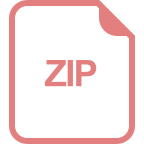
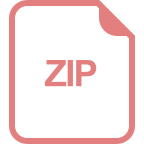
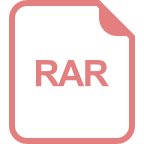
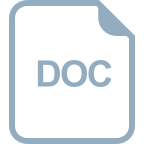
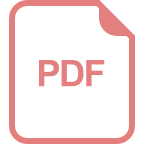
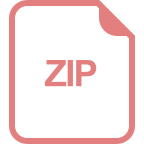
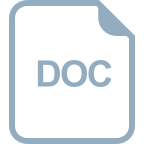
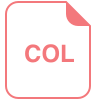


