Python实现烟花效果
时间: 2023-05-23 15:04:31 浏览: 89
A:
Python可以通过使用Pygame库实现烟花效果,下面是一个简单的演示程序:
```python
import pygame
import random
pygame.init()
# 窗口尺寸
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
# 烟花粒子类
class Particle:
def __init__(self, position, color):
self.x, self.y = position
self.color = color
self.radius = 3
self.life = 100
# 随机生成速度
speed = random.uniform(1, 5)
direction = random.uniform(0, 2 * 3.14)
self.vx = speed * pygame.math.cos(direction)
self.vy = speed * pygame.math.sin(direction)
def update(self):
self.life -= 1
self.x += self.vx
self.y += self.vy
self.vy += 0.2
self.radius = int(3 * self.life / 100)
def draw(self, screen):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
# main函数
def main():
fireworks = []
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
screen.fill((0, 0, 0))
# 生成烟花
if random.random() < 0.05:
fireworks.append(Particle((random.randint(50, width-50), height), (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))))
# 更新烟花粒子
for firework in fireworks:
firework.update()
firework.draw(screen)
if firework.life <= 0:
fireworks.remove(firework)
pygame.display.update()
if __name__ == '__main__':
main()
```
运行程序可以看到烟花效果。该程序首先定义了一个`Particle`类来表示烟花粒子,该类包括位置、颜色、生命周期、速度等信息。在`main`函数中,窗口开始循环,每隔一定时间随机生成一个烟花,并且将其添加到`fireworks`列表中。接着,程序循环更新并绘制烟花粒子。如果烟花粒子的生命周期小于或等于0,则从列表中移除。最终,通过`pygame.display.update()`函数来更新窗口画面。
阅读全文
相关推荐
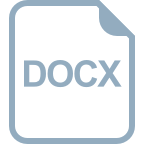

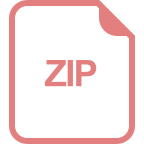
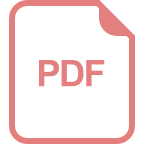
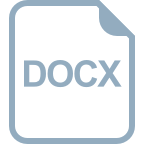
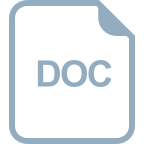
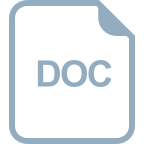
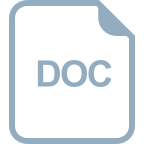
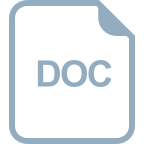
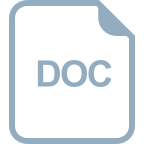
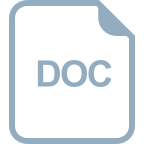
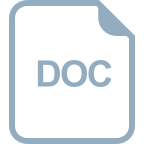
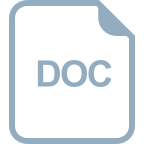
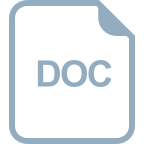
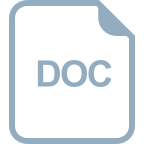
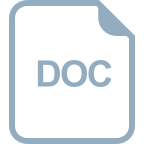