求解tsp问题,python实现,要求采用遗传算法,其中选择算子采用竞标赛选择;交叉算子采用Partial-Mapped Crossover
时间: 2024-06-10 14:06:39 浏览: 15
好的,我可以帮你解决tsp问题。遗传算法是一种常用的优化算法,它模拟了自然界的进化过程。竞标赛选择是一种选择算子,可以保留适应度高的染色体。Partial-Mapped Crossover是一种交叉算法,可以保持父母染色体的顺序,同时避免出现重复的基因。你可以参考以下示例代码实现:https://github.com/thanhhvnqb/TSP-Genetic-Algorithm-Python
相关问题
求解TSP问题,python实现,采用遗传算法其中选择算子使用竞标赛选择
好的,这个问题属于计算机科学领域,我可以回答。TSP问题是指旅行商问题,即一个旅行商需要在多个城市之间旅行,每个城市只需要访问一次,并且要求最终回到出发城市,需要找到最短的路径。遗传算法是一种常用的优化算法,在解决TSP问题中应用广泛。竞标赛选择是其中的一种选择算子,它的思想是将个体按照适应度随机分成若干个小组,每个小组中选择适应度最好的个体留下,其他个体淘汰。通过不断进行竞标赛选择,可以筛选出适应度更高的个体,从而优化求解TSP问题的结果。在Python中实现遗传算法求解TSP问题也比较简单,可以使用Python中的遗传算法库或者手动实现。
求解TSP问题,python实现,采用遗传算法其中选择算子使用竞标赛选择,请给出具体代码实现
以下是关于 TSP 问题的遗传算法实现的 Python 代码,包括竞标赛选择:
```python
import random
# 样本点列表,示例中为 5 个点
points = [(1,1), (2,3), (5,2), (7,6), (4,4)]
# 每个个体表示为一个排列,示例中为 [3, 1, 2, 4, 0]
init_population = [[i for i in range(len(points))] for j in range(10)]
# 计算代价函数,即路径总长度
def calculate_fitness(individual):
total_distance = 0
for i in range(len(individual)):
if i == len(individual) - 1:
total_distance += ((points[individual[i]][0] - points[individual[0]][0]) ** 2 + (points[individual[i]][1] - points[individual[0]][1]) ** 2) ** 0.5
else:
total_distance += ((points[individual[i]][0] - points[individual[i+1]][0]) ** 2 + (points[individual[i]][1] - points[individual[i+1]][1]) ** 2) ** 0.5
return total_distance
# 竞标赛选择算子
def tournament_selection(population, fitness_values, k):
selected_individual = None
selected_fitness = 0
for i in range(k):
index = random.randint(0, len(population)-1)
if fitness_values[index] > selected_fitness:
selected_individual = population[index]
selected_fitness = fitness_values[index]
return selected_individual
# 交叉算子,示例中使用 PMX 交叉
def pmx_crossover(parent1, parent2):
child1, child2 = parent1[:], parent2[:]
length = len(parent1)
point1 = random.randint(0, length-1)
point2 = random.randint(point1, length-1)
for i in range(point1, point2):
temp1, temp2 = child1[i], child2[i]
index1 = child1.index(temp2)
index2 = child2.index(temp1)
child1[i], child1[index1] = temp2, temp1
child2[i], child2[index2] = temp1, temp2
return child1, child2
# 变异算子,示例中使用交换变异
def swap_mutation(individual):
length = len(individual)
point1 = random.randint(0, length-1)
point2 = random.randint(0, length-1)
individual[point1], individual[point2] = individual[point2], individual[point1]
return individual
# 遗传算法主函数
def genetic_algorithm(population, fitness_func, tournament_size, crossover_rate, mutation_rate, max_generations):
for i in range(max_generations):
# 评估种群
fitness_values = [fitness_func(individual) for individual in population]
# 找出最优个体
best_index = fitness_values.index(min(fitness_values))
best_individual = population[best_index]
# 输出每代最优个体的路径长度
print('Generation {}: {}'.format(i+1, calculate_fitness(best_individual)))
# 进行竞标赛选择,生成新的子代种群
new_population = []
while len(new_population) < len(population):
parent1 = tournament_selection(population, fitness_values, tournament_size)
parent2 = tournament_selection(population, fitness_values, tournament_size)
if random.uniform(0, 1) < crossover_rate:
child1, child2 = pmx_crossover(parent1, parent2)
new_population.append(child1)
new_population.append(child2)
else:
new_population.append(parent1)
new_population.append(parent2)
# 进行变异操作
for j in range(len(new_population)):
if random.uniform(0, 1) < mutation_rate:
new_population[j] = swap_mutation(new_population[j])
# 更新种群
population = new_population
return population[best_index]
# 运行遗传算法
best_path = genetic_algorithm(init_population, calculate_fitness, 3, 0.8, 0.01, 10)
print('Best path: {}'.format(best_path))
```
其中,样本点列表 `points` 表示所有的点坐标,例如 `(1,1)` 表示横坐标为 1,纵坐标为 1 的点。`init_population` 是种群的初始化列表,其中每个个体表示为一个排列。
`calculate_fitness` 函数计算一个个体的代价函数,本例中使用的是路径总长度。`tournament_selection` 是竞标赛选择算子。`pmx_crossover` 是 PMX 交叉算子,交叉率为 `crossover_rate`。`swap_mutation` 是交换变异算子,变异率为 `mutation_rate`。
`genetic_algorithm` 是遗传算法的主函数,其中的参数包括竞标赛选择算子的比赛规模 `tournament_size`、交叉率 `crossover_rate`、变异率 `mutation_rate`、最大迭代次数 `max_generations` 等。其中,每代种群的最优个体的路径长度会被输出,最终的最优路径会被返回。
使用以上代码运行 TSP 问题的遗传算法,可以得到求解结果。
相关推荐
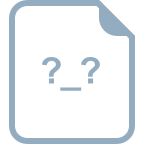
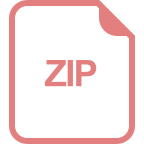
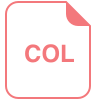











