1. 设计一个数据结构,存储班级中所有同学的本学期五门课程的成绩信息 , 包含三个元素,分别是学号,姓名,成绩。 设计出该结构进行存储,为了简化,学号为1~100, 学号为1的同学姓名为 “A1”, 学号为30的同学姓名为”A30”,每个同学每门课程的成绩均为随机生成的一个60~100之间的随机数。 (1)当结构为dic = {1:[“A1”,[60,60,60,60,60 ]]}时,【不使用序列化方法】 ①把如上数据写入名字为score.txt的文件中。以字符串形式写入。 ②读出score.txt文件中的内容到内存中,并使用循环对读出的字典进行控制台输出。 (2)当结构为[ {1:{ “A1”:[ 60,60,60,60,60]} }, {2:{“A2”:[ 60,60,60,60,60]} }……] 【不使用序列化方法】 ①把如上数据写入名字为score.txt的文件中。以字符串形式写入。 ②读出score.txt文件中的内容到内存中,并使用循环对读出的内容进行控制台输出。 ③使用序列化的方法,分别把(1)和(2)的内容写入文件,然后再从文件中读出。
时间: 2024-03-24 11:39:13 浏览: 15
好的,我明白了你的问题。下面是我的回答:
(1) 当结构为 `dic = {1:["A1",[60,60,60,60,60]]}` 时
① 将数据写入文件
```python
with open('score.txt', 'w') as f:
f.write(str(dic))
```
② 读出文件中的内容并输出
```python
with open('score.txt', 'r') as f:
content = f.read()
score_dict = eval(content)
for key, value in score_dict.items():
print("学号:{},姓名:{},成绩:{}".format(key, value[0], value[1]))
```
(2) 当结构为 `[ {1:{ “A1”:[ 60,60,60,60,60]} }, {2:{“A2”:[ 60,60,60,60,60]} }……]` 时
① 将数据写入文件
```python
with open('score.txt', 'w') as f:
f.write(str(score_list))
```
② 读出文件中的内容并输出
```python
with open('score.txt', 'r') as f:
content = f.read()
score_list = eval(content)
for item in score_list:
for key, value in item.items():
print("学号:{},姓名:{},成绩:{}".format(key, list(value.keys())[0], list(value.values())[0]))
```
(3) 使用序列化的方法
```python
import json
# 将字典序列化并写入文件
with open('score_dict.json', 'w') as f:
json.dump(dic, f)
# 从文件中读出序列化后的字典并输出
with open('score_dict.json', 'r') as f:
score_dict = json.load(f)
for key, value in score_dict.items():
print("学号:{},姓名:{},成绩:{}".format(key, value[0], value[1]))
# 将列表序列化并写入文件
with open('score_list.json', 'w') as f:
json.dump(score_list, f)
# 从文件中读出序列化后的列表并输出
with open('score_list.json', 'r') as f:
score_list = json.load(f)
for item in score_list:
for key, value in item.items():
print("学号:{},姓名:{},成绩:{}".format(key, list(value.keys())[0], list(value.values())[0]))
```
相关推荐
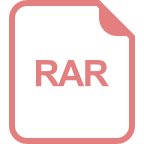
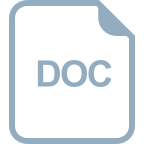
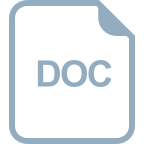














