基于c语言的ecc实现方法
时间: 2024-05-11 15:20:26 浏览: 25
实现椭圆曲线加密算法(ECC)的基本步骤如下:
1.定义椭圆曲线参数a、b、p、G、n,其中a、b、p分别为椭圆曲线的参数,G为基点,n为G的阶。
2.选择一个私钥d,并计算公钥Q=dG。
3.选择一个随机数k,并计算点P=kG。
4.将点P的x坐标和y坐标作为密文发送给接收方。
5.接收方使用自己的私钥d计算点Q=dG,并将点P乘以d得到点Q=Pd。
6.接收方使用点Q的x坐标和y坐标还原出点P,即P=Qd^-1。
下面是一个基于C语言的椭圆曲线加密算法(ECC)实现方法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/ec.h>
#include <openssl/ecdsa.h>
#include <openssl/objects.h>
int main()
{
EC_KEY *eckey = NULL;
EC_GROUP *group = NULL;
EC_POINT *point = NULL;
BIGNUM *bn_d = NULL;
BIGNUM *bn_k = NULL;
const EC_POINT *pubkey = NULL;
unsigned char *msg = "hello world";
unsigned char *sig = NULL;
size_t siglen = 0;
int ret = 0;
// 选择椭圆曲线参数
group = EC_GROUP_new_by_curve_name(NID_secp256k1);
if (group == NULL) {
fprintf(stderr, "EC_GROUP_new_by_curve_name error\n");
goto exit;
}
// 生成密钥对
eckey = EC_KEY_new();
if (eckey == NULL) {
fprintf(stderr, "EC_KEY_new error\n");
goto exit;
}
ret = EC_KEY_set_group(eckey, group);
if (ret != 1) {
fprintf(stderr, "EC_KEY_set_group error\n");
goto exit;
}
ret = EC_KEY_generate_key(eckey);
if (ret != 1) {
fprintf(stderr, "EC_KEY_generate_key error\n");
goto exit;
}
// 获取私钥
bn_d = EC_KEY_get0_private_key(eckey);
// 获取公钥
point = EC_KEY_get0_public_key(eckey);
if (point == NULL) {
fprintf(stderr, "EC_KEY_get0_public_key error\n");
goto exit;
}
pubkey = point;
// 加密
bn_k = BN_new();
if (bn_k == NULL) {
fprintf(stderr, "BN_new error\n");
goto exit;
}
ret = BN_pseudo_rand(bn_k, 256, 0, 0);
if (ret != 1) {
fprintf(stderr, "BN_pseudo_rand error\n");
goto exit;
}
point = EC_POINT_new(group);
if (point == NULL) {
fprintf(stderr, "EC_POINT_new error\n");
goto exit;
}
ret = EC_POINT_mul(group, point, bn_k, NULL, NULL, NULL);
if (ret != 1) {
fprintf(stderr, "EC_POINT_mul error\n");
goto exit;
}
siglen = EC_POINT_point2oct(group, point, POINT_CONVERSION_UNCOMPRESSED, NULL, 0, NULL);
sig = (unsigned char *)malloc(siglen);
if (sig == NULL) {
fprintf(stderr, "malloc error\n");
goto exit;
}
ret = EC_POINT_point2oct(group, point, POINT_CONVERSION_UNCOMPRESSED, sig, siglen, NULL);
if (ret != siglen) {
fprintf(stderr, "EC_POINT_point2oct error\n");
goto exit;
}
// 解密
point = EC_POINT_new(group);
if (point == NULL) {
fprintf(stderr, "EC_POINT_new error\n");
goto exit;
}
ret = EC_POINT_oct2point(group, point, sig, siglen, NULL);
if (ret != 1) {
fprintf(stderr, "EC_POINT_oct2point error\n");
goto exit;
}
point = EC_POINT_mul(group, NULL, bn_d, point, NULL, NULL);
if (point == NULL) {
fprintf(stderr, "EC_POINT_mul error\n");
goto exit;
}
siglen = EC_POINT_point2oct(group, point, POINT_CONVERSION_UNCOMPRESSED, NULL, 0, NULL);
sig = (unsigned char *)realloc(sig, siglen);
if (sig == NULL) {
fprintf(stderr, "realloc error\n");
goto exit;
}
ret = EC_POINT_point2oct(group, point, POINT_CONVERSION_UNCOMPRESSED, sig, siglen, NULL);
if (ret != siglen) {
fprintf(stderr, "EC_POINT_point2oct error\n");
goto exit;
}
printf("msg=%s, siglen=%zu, sig=%s\n", msg, siglen, sig);
exit:
if (sig != NULL) {
free(sig);
}
if (bn_k != NULL) {
BN_free(bn_k);
}
if (bn_d != NULL) {
BN_free(bn_d);
}
if (point != NULL) {
EC_POINT_free(point);
}
if (eckey != NULL) {
EC_KEY_free(eckey);
}
if (group != NULL) {
EC_GROUP_free(group);
}
return 0;
}
```
该示例代码使用了OpenSSL库中的EC_KEY、EC_GROUP、EC_POINT等数据结构和函数来实现椭圆曲线加密算法(ECC)。在示例代码中,我们使用secp256k1椭圆曲线参数生成了一个密钥对,并使用随机数k加密了一段消息,然后使用私钥d解密了该消息。在实际应用中,我们需要根据具体的情况选择合适的椭圆曲线参数,并采取适当的措施来保护私钥的安全。
相关推荐
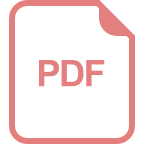
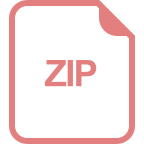
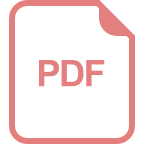
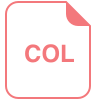
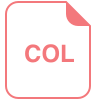
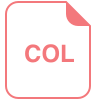
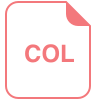
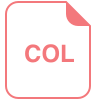










