ts循环数组
时间: 2023-07-31 22:05:30 浏览: 57
循环数组也叫环形数组,是一种特殊的数组,它的最后一个元素与第一个元素相邻,形成一个环,因此可以循环遍历。在 TypeScript 中实现循环数组,可以使用以下代码:
```
class CircularArray<T> {
private data: T[];
private readIndex: number;
private writeIndex: number;
constructor(length: number) {
this.data = new Array(length);
this.readIndex = 0;
this.writeIndex = 0;
}
public enqueue(item: T) {
if (this.isFull()) {
throw new Error("Circular array is full.");
}
this.data[this.writeIndex] = item;
this.writeIndex = (this.writeIndex + 1) % this.data.length;
}
public dequeue(): T {
if (this.isEmpty()) {
throw new Error("Circular array is empty.");
}
const item = this.data[this.readIndex];
this.readIndex = (this.readIndex + 1) % this.data.length;
return item;
}
public isEmpty(): boolean {
return this.readIndex === this.writeIndex;
}
public isFull(): boolean {
return (this.writeIndex + 1) % this.data.length === this.readIndex;
}
}
```
这个实现包括了队列的基本操作,enqueue 和 dequeue,以及判断循环数组是否为空和是否已满的方法 isEmpty 和 isFull。需要注意的是,循环数组的大小在初始化时就已经被确定,因此无法改变。
相关推荐
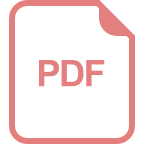
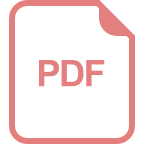














