python随机加减法图形界面
时间: 2023-10-13 12:05:02 浏览: 49
以下是一个简单的 Python 图形界面程序,可以生成随机的加减法题目,并且计算出答案。你可以使用 tkinter 库来实现这个程序。
```python
import random
import tkinter as tk
class MathGame:
def __init__(self, master):
self.master = master
master.title("Math Game")
self.num1 = random.randint(0, 99)
self.num2 = random.randint(0, 99)
self.operation = random.choice(["+", "-"])
self.answer = self.calculate()
self.question_label = tk.Label(master, text=f"{self.num1} {self.operation} {self.num2} = ")
self.question_label.pack()
self.answer_entry = tk.Entry(master)
self.answer_entry.pack()
self.check_button = tk.Button(master, text="Check", command=self.check_answer)
self.check_button.pack()
def calculate(self):
if self.operation == "+":
return self.num1 + self.num2
elif self.operation == "-":
return self.num1 - self.num2
def check_answer(self):
answer = int(self.answer_entry.get())
if answer == self.answer:
self.question_label.config(text="Correct!")
else:
self.question_label.config(text="Incorrect!")
self.num1 = random.randint(0, 99)
self.num2 = random.randint(0, 99)
self.operation = random.choice(["+", "-"])
self.answer = self.calculate()
self.answer_entry.delete(0, tk.END)
self.question_label.config(text=f"{self.num1} {self.operation} {self.num2} = ")
root = tk.Tk()
game = MathGame(root)
root.mainloop()
```
在上面的代码中,我们首先定义了一个名为 `MathGame` 的类,它是我们的主要程序。在 `__init__` 方法中,我们随机生成两个数字和一个加减号,然后计算出答案。我们使用 `tk.Label` 来显示题目, `tk.Entry` 来接收用户输入的答案,然后使用 `tk.Button` 来检查答案。当用户点击“Check”按钮时,我们将检查用户的答案是否正确,并根据结果更新题目和答案。
最后,我们创建一个 `tk.Tk()` 对象并将其传递给 `MathGame` 类,然后调用 `root.mainloop()` 来启动程序的图形界面。运行程序后,你应该可以看到一个简单的界面,可以生成随机的加减法题目。
相关推荐
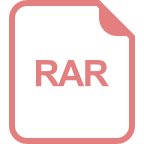
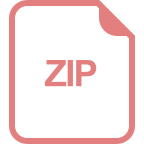
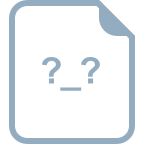














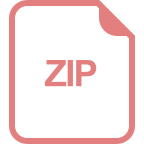