Java opencv ArrayList<Point> 线性回归拟合直线
时间: 2023-07-09 14:05:00 浏览: 48
你可以使用Java OpenCV中的Polyfit函数来进行线性回归拟合直线。该函数可以通过输入一系列的数据点,返回一条最优拟合直线的参数。
以下是一个使用Polyfit函数进行线性回归拟合直线的示例代码:
```java
import org.opencv.core.*;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import java.util.ArrayList;
import java.util.List;
public class LinearRegressionExample {
public static void main(String[] args) {
// Load image
Mat image = Imgcodecs.imread("path/to/image.jpg");
// Convert to grayscale
Mat gray = new Mat();
Imgproc.cvtColor(image, gray, Imgproc.COLOR_BGR2GRAY);
// Detect edges
Mat edges = new Mat();
Imgproc.Canny(gray, edges, 50, 200);
// Find contours
List<MatOfPoint> contours = new ArrayList<>();
Imgproc.findContours(edges, contours, new Mat(), Imgproc.RETR_LIST, Imgproc.CHAIN_APPROX_SIMPLE);
// Fit lines to contours
for (MatOfPoint contour : contours) {
double[] coefficients = new double[2];
MatOfPoint2f curve = new MatOfPoint2f(contour.toArray());
Imgproc.fitLine(curve, new Mat(), Imgproc.DIST_L2, 0, 0.01, 0.01, coefficients);
double k = coefficients[1] / coefficients[0];
double b = coefficients[3] - k * coefficients[2];
System.out.println("Line equation: y = " + k + "x + " + b);
}
}
}
```
在上面的代码中,我们首先将图像转换为灰度图像,并使用Canny算法检测边缘。然后,我们使用findContours函数查找所有轮廓,并对每个轮廓使用fitLine函数进行线性回归拟合。最后,我们输出每条直线的方程。
注意,Polyfit函数返回的参数是一个数组,其中包含拟合直线的斜率和截距。在上面的代码中,我们计算了斜率和截距,并输出了线性方程。
相关推荐
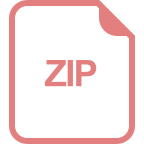















