python fastapi 定义一个html页面。服务端返回excel文件内容,网页提供编辑功能
时间: 2024-09-19 15:18:13 浏览: 56
在Python FastAPI项目中,你可以结合HTML模板和FastAPI的响应流(Streaming Response)来创建一个允许用户下载Excel文件并提供基础编辑功能的界面。以下是一个简单的步骤指南:
1. **安装依赖库**:
首先,你需要安装`fastapi`, `jinja2`(用于渲染HTML模板)以及`pandas`或`openpyxl`等处理Excel数据的库。
```shell
pip install fastapi jinja2 pandas openpyxl
```
2. **设置FastAPI应用**:
创建一个FastAPI应用,并定义一个路由来返回Excel文件。
```python
from fastapi import FastAPI, File, StreamingResponse, UploadFile
import pandas as pd
app = FastAPI()
@app.post("/download_excel")
async def download_excel(file: UploadFile = File(...)):
# 读取Excel内容
df = pd.read_excel(file.file)
# 创建Excel响应
response = StreamingResponse(
df.to_excel(StringIO(), engine="openpyxl", index=False),
media_type="application/vnd.openxmlformats-officedocument.spreadsheetml.sheet",
headers={"Content-Disposition": "attachment; filename=report.xlsx"}
)
return response
```
这里,`upload_excel`函数接收上传的Excel文件作为输入,然后将其内容转换为Excel流,并设置合适的头信息让浏览器识别它为需要下载的文件。
3. **创建HTML模板**:
使用Jinja2模板来创建一个HTML页面,允许用户选择文件并触发下载。例如,在`templates/download.html`:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Download Excel</title>
</head>
<body>
<h1>Select an Excel file to download:</h1>
<form action="/download_excel" method="post" enctype="multipart/form-data">
<input type="file" name="file" accept=".xlsx, .xls">
<button type="submit">Upload and Download</button>
</form>
</body>
</html>
```
4. **启动服务器**:
确保FastAPI应用配置好后,运行服务器:
```python
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
```
现在,当你访问`http://localhost:8000/download_excel`并选择一个Excel文件上传后,服务器会生成并返回该文件。对于编辑功能,由于HTTP请求通常是只读的,你可以考虑前端JavaScript配合一些在线Excel编辑库(如SheetJS或Google Sheets API),但这超出了FastAPI本身的功能范围。
阅读全文
相关推荐
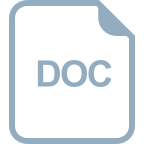
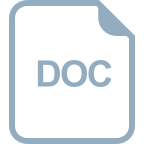
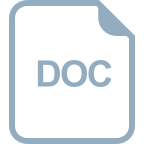
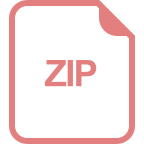
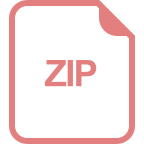
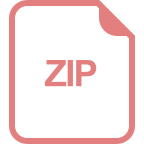
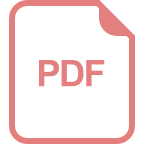
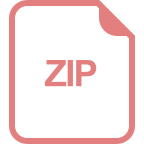
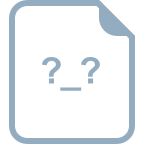
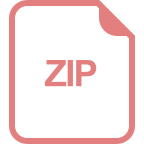
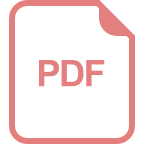
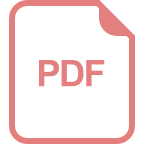
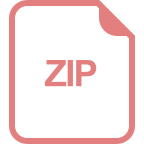
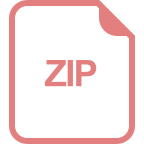
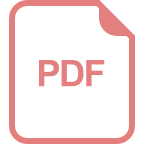
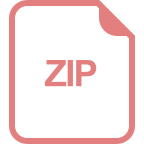
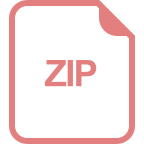