帮助编写多车辆单配送中心的VRP问题的代码 问题描述: 烟草物流作为特殊的行业物流,其卷烟的配送路径问题是一种大规模车辆路径问题,使车辆从配送中心出发,配送至各个聚类中心服务站点,完成用户需求返回配送中心,问题是规划车辆的配送路径,优化目标是如何构造适当的路径,使得在满足一定的约束条件下,完成全部需求花费的总成本最小(总利润最大)。配送线路优化问题可界定为单调度中心、多车型,非满载、带最大配送里程约束的物流配送线路优化问题。 约束条件: 1)所有车辆均从配送中心出发,完成配送作业后空车返回配送中心; (2)卷烟配送中心负责所有客户的配送业务,且配送中心和零售户的位置 已知; (3)配送中心内有多种车型,其中车辆的型号、各车型容积及其最大载货 量已知; (4)每个区域内的客户只能由一种类型的车进行服务,且只能被访问一次; (5)各区域内不同客户订购的卷烟可混合装车,但区域内的客户总需求量 不得超过该车的最大装载量; 6)配送过程中客户数量、需求量等订单信息已知; (7)配送人员在正常工作时间内最大为 6 小时; (8)配送过程中无突发情况发生,天气、道路状况、车辆运行均正常。
时间: 2024-03-31 08:37:17 浏览: 31
由于多车辆单配送中心的VRP问题非常复杂,需要考虑多个因素,因此代码实现比较复杂。以下是一个基本的Python代码示例,可以实现部分功能:
```python
import random
import math
class Customer:
def __init__(self, x, y, demand):
self.x = x
self.y = y
self.demand = demand
class Vehicle:
def __init__(self, capacity):
self.capacity = capacity
self.route = []
class VRP:
def __init__(self, depot, customers, vehicles):
self.depot = depot
self.customers = customers
self.vehicles = vehicles
self.distance_matrix = self.calculate_distance_matrix()
def calculate_distance_matrix(self):
matrix = []
for i in range(len(self.customers) + 1):
row = []
for j in range(len(self.customers) + 1):
if i == j:
row.append(0)
elif i == 0:
row.append(math.sqrt((self.depot.x - self.customers[j-1].x)**2 + (self.depot.y - self.customers[j-1].y)**2))
elif j == 0:
row.append(math.sqrt((self.depot.x - self.customers[i-1].x)**2 + (self.depot.y - self.customers[i-1].y)**2))
else:
row.append(math.sqrt((self.customers[i-1].x - self.customers[j-1].x)**2 + (self.customers[i-1].y - self.customers[j-1].y)**2))
matrix.append(row)
return matrix
def get_route_cost(self, route):
cost = 0
for i in range(len(route) - 1):
cost += self.distance_matrix[route[i]][route[i+1]]
return cost
def get_total_cost(self):
total_cost = 0
for vehicle in self.vehicles:
total_cost += self.get_route_cost(vehicle.route)
return total_cost
def get_feasible_routes(self, vehicle, remaining_customers):
feasible_routes = []
for k in range(len(remaining_customers)):
if remaining_customers[k].demand <= vehicle.capacity:
new_route = vehicle.route[:]
new_route.append(remaining_customers[k])
new_vehicle = Vehicle(vehicle.capacity - remaining_customers[k].demand)
new_vehicle.route = new_route
new_remaining_customers = remaining_customers[:k] + remaining_customers[k+1:]
if len(new_remaining_customers) == 0:
feasible_routes.append([new_vehicle])
else:
feasible_routes += [[new_vehicle] + r for r in self.get_feasible_routes(new_vehicle, new_remaining_customers)]
return feasible_routes
def solve(self):
remaining_customers = self.customers[:]
for vehicle in self.vehicles:
feasible_routes = self.get_feasible_routes(vehicle, remaining_customers)
if len(feasible_routes) == 0:
break
route_costs = [self.get_route_cost(route) for route in feasible_routes]
min_cost = min(route_costs)
min_index = route_costs.index(min_cost)
vehicle.route = feasible_routes[min_index][0].route
remaining_customers = feasible_routes[min_index][1:] + remaining_customers
return self.get_total_cost()
# example usage
depot = Customer(0, 0, 0)
customers = [Customer(random.random() * 100, random.random() * 100, random.randint(1, 10)) for i in range(20)]
vehicles = [Vehicle(20), Vehicle(30)]
vrp = VRP(depot, customers, vehicles)
cost = vrp.solve()
print(cost)
```
这段代码实现了一个简单的VRP问题,其中包括了顾客和车辆的定义,距离矩阵的计算,路径成本的计算,可行路径的搜索等功能。由于VRP问题的复杂性较高,该代码只能解决一些简单的问题,对于较复杂的问题需要进行更加细致的编码。
相关推荐
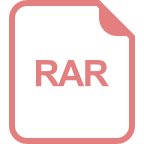
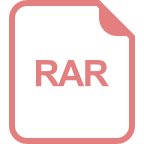
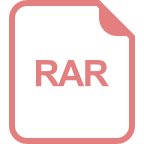














