具体怎么用代码实现用DQN完成BipedalWalkerHardcore_v3环境
时间: 2024-02-06 21:10:16 浏览: 107
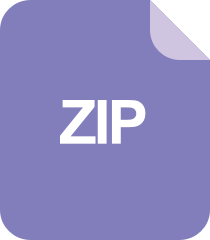
SimulinkPendulumDQN_pendulum_SIMULINK_MATLAB实现DQN_DQN_DQNmatlab_
使用DQN算法来解决BipedalWalkerHardcore_v3环境需要进行以下步骤:
1. 安装 gym 和 pytorch 库
```python
pip install gym torch
```
2. 定义神经网络模型
在这里我们使用一个简单的三层全连接神经网络作为模型。
```python
import torch.nn as nn
import torch.nn.functional as F
class DQN(nn.Module):
def __init__(self, input_shape, num_actions):
super(DQN, self).__init__()
self.fc1 = nn.Linear(input_shape, 128)
self.fc2 = nn.Linear(128, 128)
self.fc3 = nn.Linear(128, num_actions)
def forward(self, x):
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
```
3. 定义DQN算法
我们使用经典的DQN算法来训练模型。DQN算法中使用了一个经验回放缓存区来存储历史经验,然后从缓存区中随机采样一批数据进行训练。
```python
import random
import numpy as np
import torch.optim as optim
import torch.nn.functional as F
class DQNAgent:
def __init__(self, state_shape, num_actions, learning_rate, gamma, epsilon_start, epsilon_end, epsilon_decay):
self.device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
self.state_shape = state_shape
self.num_actions = num_actions
self.learning_rate = learning_rate
self.gamma = gamma
self.epsilon = epsilon_start
self.epsilon_end = epsilon_end
self.epsilon_decay = epsilon_decay
self.model = DQN(state_shape, num_actions).to(self.device)
self.target_model = DQN(state_shape, num_actions).to(self.device)
self.optimizer = optim.Adam(self.model.parameters(), lr=learning_rate)
self.memory = []
def act(self, state):
if random.random() < self.epsilon:
return random.randint(0, self.num_actions - 1)
else:
state = torch.FloatTensor(state).unsqueeze(0).to(self
阅读全文
相关推荐
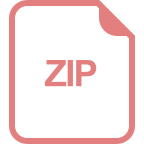
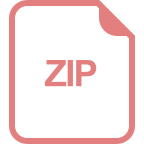
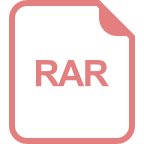
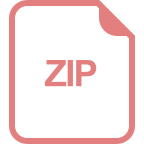
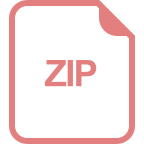
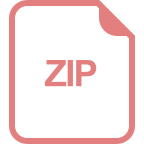
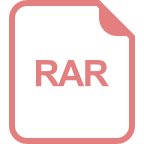
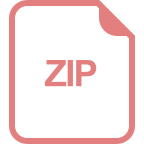
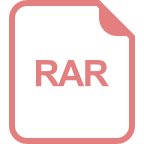
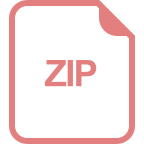
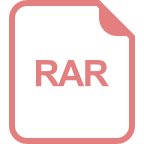
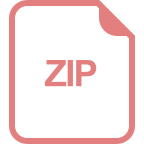
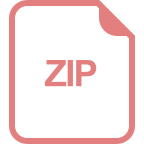
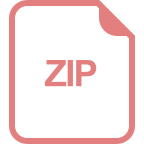