写一个Django文件上传与下载模块
时间: 2023-08-31 07:36:38 浏览: 89
### 回答1:
我可以为你提供一个示例:首先,在你的Django项目中,新建一个应用,让它能够处理文件上传与下载。然后,在你的应用中新建一个模型。模型应该包括一个文件字段,用于存储上传的文件。接着,建立一个视图,来实现文件的上传和下载功能。最后,添加一个urls文件,用于设置文件上传与下载的路由。这样,你就可以创建一个完整的Django文件上传与下载模块了。
### 回答2:
在Django中,可以通过使用django.views.decorators.csrf装饰器来保护文件上传和下载功能,以防止跨站请求伪造。下面是一个简单的Django文件上传与下载模块的示例:
1.首先,在Django的settings.py文件中配置MEDIA_ROOT和MEDIA_URL:
```python
MEDIA_ROOT = os.path.join(BASE_DIR, 'media/')
MEDIA_URL = '/media/'
```
2.创建一个视图函数来处理文件上传请求:
```python
from django.shortcuts import render
def file_upload(request):
if request.method == 'POST':
uploaded_file = request.FILES['file']
fs = FileSystemStorage()
fs.save(uploaded_file.name, uploaded_file)
return render(request, 'upload_success.html')
return render(request, 'file_upload.html')
```
3.创建一个模板文件来显示文件上传表单(file_upload.html):
```html
<form method="post" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="file">
<button type="submit">上传</button>
</form>
```
4.创建一个模板文件来显示文件上传成功的消息(upload_success.html):
```html
<h1>文件上传成功!</h1>
```
5.配置一个URL模式来调用file_upload视图函数:
```python
from django.urls import path
from .views import file_upload
urlpatterns = [
path('upload/', file_upload, name='file_upload'),
]
```
现在,用户可以通过访问`/upload/`来上传文件。
6.创建一个视图函数来处理文件下载请求:
```python
from django.http import HttpResponse, Http404
from django.conf import settings
import os
def file_download(request, file_name):
file_path = os.path.join(settings.MEDIA_ROOT, file_name)
if os.path.exists(file_path):
with open(file_path, 'rb') as fh:
response = HttpResponse(fh.read(), content_type="application/octet-stream")
response['Content-Disposition'] = 'attachment; filename=' + os.path.basename(file_path)
return response
raise Http404
```
7.配置一个URL模式来调用file_download视图函数:
```python
from django.urls import path
from .views import file_download
urlpatterns = [
path('download/<str:file_name>/', file_download, name='file_download'),
]
```
现在,用户可以通过访问`/download/文件名/`来下载文件。
### 回答3:
Django是一个开发Web应用的Python框架,可以轻松实现文件上传和下载功能。下面是一个简单的Django文件上传与下载模块的实现:
1. 首先,需要在Django项目的settings.py文件中配置文件存储路径。可以设置MEDIA_ROOT来指定文件上传的目录:
```
MEDIA_ROOT = os.path.join(BASE_DIR, 'media')
```
2. 在项目的urls.py文件中配置文件上传和下载的路由:
```
from django.conf.urls.static import static
from django.conf import settings
urlpatterns = [
# 其他路由配置
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
```
3. 在Django的views.py文件中实现文件上传和下载的处理逻辑。以下是一个简单的示例:
文件上传:
```python
from django.shortcuts import render
from django.core.files.storage import FileSystemStorage
def upload_file(request):
if request.method == 'POST' and request.FILES['file']:
file = request.FILES['file']
fs = FileSystemStorage()
filename = fs.save(file.name, file)
file_url = fs.url(filename)
return render(request, 'upload.html', {'file_url': file_url})
return render(request, 'upload.html')
```
文件下载:
```python
from django.http import FileResponse
from django.core.files.storage import FileSystemStorage
def download_file(request, filename):
fs = FileSystemStorage()
file_path = fs.path(filename)
file = open(file_path, 'rb')
response = FileResponse(file)
response['Content-Disposition'] = 'attachment; filename="{}"'.format(filename)
return response
```
4. 在Django的templates文件夹中创建upload.html文件,用于上传文件展示和下载链接的页面:
```html
<form method="post" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="file">
<button type="submit">上传</button>
</form>
{% if file_url %}
<a href="{{ file_url }}">下载文件</a>
{% endif %}
```
以上就是一个简单的Django文件上传与下载模块的实现。根据项目需求,可能需要进一步优化和扩展该模块,例如增加文件类型验证、文件大小限制等功能。
相关推荐
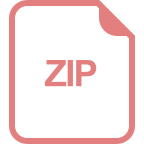
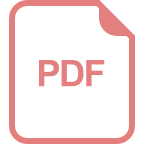
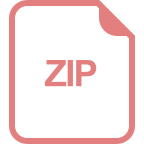














