空白页检测python程序
时间: 2023-08-25 18:06:10 浏览: 64
空白页检测的 Python 程序可以通过以下步骤实现:
1. 导入必要的库,比如 os 和 re
2. 定义一个函数,接收一个文件路径作为输入
3. 使用 os.path.getsize() 函数检查文件大小是否为 0,如果是则返回 True,否则继续执行
4. 打开文件,使用 re 模块的 search() 方法搜索空白符,如果找到则返回 False,否则返回 True
5. 关闭文件,函数结束
下面是一个示例程序:
```python
import os
import re
def is_blank(file_path):
if os.path.getsize(file_path) == 0:
return True
else:
with open(file_path, 'r') as f:
content = f.read()
if re.search('\S', content):
return False
else:
return True
```
你可以调用这个函数并传入需要检测的文件路径,它会返回 True 或 False,表示文件是否为空白页。
相关问题
写一个pdf空白图片检测python程序,返回文件名和页数
PDF 空白页检测的 Python 程序可以通过以下步骤实现:
1. 导入必要的库,比如 PyPDF2、Pillow 和 numpy
2. 定义一个函数,接收一个 PDF 文件路径作为输入
3. 使用 PyPDF2 的 PdfFileReader() 函数打开 PDF 文件,并获取其页数
4. 遍历每一页,使用 Pillow 的 Image.frombytes() 函数将其转换为 numpy 数组
5. 计算数组中所有像素的平均值,如果平均值接近于 255(白色),则说明该页为空白页,将该页页码添加到一个空白数组中
6. 返回所有空白页的页码以及文件名
下面是一个示例程序:
```python
import PyPDF2
from PIL import Image
import numpy as np
def detect_blank_pages(pdf_path):
pdf_file = open(pdf_path, 'rb')
pdf_reader = PyPDF2.PdfFileReader(pdf_file)
num_pages = pdf_reader.getNumPages()
blank_pages = []
for page_num in range(num_pages):
page = pdf_reader.getPage(page_num)
page_content = page['/Contents'].getData()
if page_content:
img = np.array(Image.frombytes('RGB', (page.mediaBox.getWidth(), page.mediaBox.getHeight()), page_content))
if np.mean(img) > 250:
blank_pages.append(page_num + 1)
pdf_file.close()
return pdf_path, blank_pages
```
你可以调用这个函数并传入需要检测的 PDF 文件路径,它会返回一个包含文件名和空白页页码的元组。需要注意的是,这个程序只能检测单色 PDF,对于彩色 PDF 需要做相应的处理。
写一个检测pdf是否是空白页的python程序,判断是空白页有两个条件:1.pdf没有任何元素2.pdf有图片但是图片为空白,返回空白页的文件名和第几页数
好的,以下是一个检测pdf是否是空白页的Python程序,使用了PyPDF2和Pillow库:
```python
import PyPDF2
from PIL import Image
def is_blank_page(pdf_path, page_num):
pdf_file = open(pdf_path, 'rb')
pdf_reader = PyPDF2.PdfFileReader(pdf_file)
page = pdf_reader.getPage(page_num)
# Check if page has no elements
if page['/Contents'] is None:
return True
# Check if page has blank image
for obj in page['/Resources']['/XObject'].values():
if obj['/Subtype'] == '/Image':
img = PyPDF2.PdfImageXObject(obj)
img_data = img.getData()
if Image.open(img_data).getbbox() is not None:
return False
return True
# Example usage
pdf_path = 'example.pdf'
for i in range(10):
if is_blank_page(pdf_path, i):
print(f'Page {i+1} of {pdf_path} is a blank page')
```
这个程序会打开指定的pdf文件,然后检查每一页是否为空白页。如果某一页没有任何元素,或者存在图片但是图片为空白,那么就认为这一页是空白页。程序会输出每个空白页的页码和pdf文件名。你可以根据需要修改代码,比如更改输出格式等等。
相关推荐
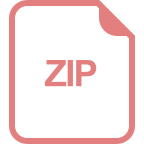






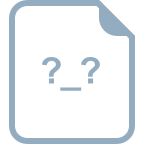
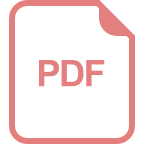
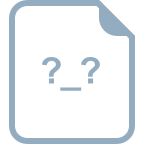