python使用线程
时间: 2024-05-24 07:07:35 浏览: 11
Python的线程模块`threading`可以用来创建和管理线程。在Python中,线程的创建和管理相对于其他语言来说是比较简单的。以下是使用Python的线程模块`threading`来实现多线程的一些基本步骤:
1. 导入`threading`模块
```
import threading
```
2. 定义线程函数,即需要线程执行的任务
```
def thread_function(arg1, arg2, ...):
# 执行任务
...
```
3. 创建线程对象,传入线程函数和参数
```
thread = threading.Thread(target=thread_function, args=(arg1, arg2, ...))
```
4. 启动线程
```
thread.start()
```
5. 等待线程执行完成
```
thread.join()
```
6. 如果有多个线程需要执行,则重复步骤3-5
下面是一个简单的例子,演示了如何使用Python的线程模块`threading`来实现多线程:
```
import threading
import time
def thread_function(thread_id, sleep_time):
print(f"Thread {thread_id} started")
time.sleep(sleep_time)
print(f"Thread {thread_id} finished")
threads = []
for i in range(5):
thread = threading.Thread(target=thread_function, args=(i, i+1))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print("All threads finished")
```
运行结果如下:
```
Thread 0 started
Thread 1 started
Thread 2 started
Thread 3 started
Thread 4 started
Thread 0 finished
Thread 1 finished
Thread 2 finished
Thread 3 finished
Thread 4 finished
All threads finished
```
相关推荐
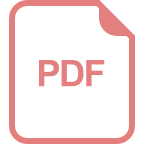
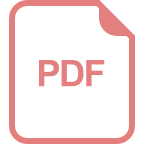
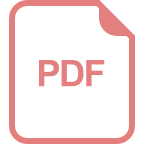
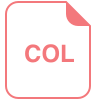
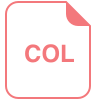
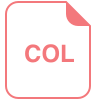
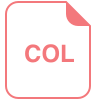
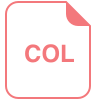









