使用vs的string的split函数进行分割字符串
时间: 2023-07-15 10:10:36 浏览: 295
在 C++ 中,可以使用 `std::string` 的 `find` 和 `substr` 函数来实现字符串的分割。可以通过循环查找分隔符,并将子字符串从原始字符串中提取出来。代码如下:
```cpp
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string str = "Hello,World,How,Are,You";
char delimiter = ',';
std::vector<std::string> tokens;
size_t pos = 0;
std::string token;
while ((pos = str.find(delimiter)) != std::string::npos) {
token = str.substr(0, pos);
tokens.push_back(token);
str.erase(0, pos + 1);
}
tokens.push_back(str);
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
输出结果为:
```
Hello
World
How
Are
You
```
如果你使用的是 Visual Studio,也可以使用 `std::string` 的 `split` 函数来进行分割。这个函数是 C++17 中新增的,但是 Visual Studio 2019 已经支持了。使用方法如下:
```cpp
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string str = "Hello,World,How,Are,You";
char delimiter = ',';
std::vector<std::string> tokens;
std::string token;
for (const auto& t : str | std::views::split(delimiter)) {
token = std::string(t.begin(), t.end());
tokens.push_back(token);
}
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
输出结果与上面的代码相同。需要注意的是,使用 `split` 函数需要包含头文件 `<ranges>`。
阅读全文
相关推荐
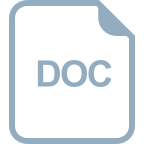
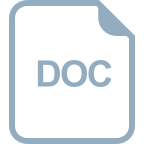
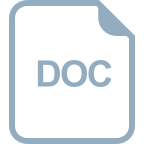
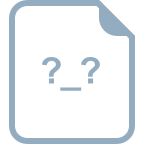
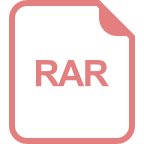
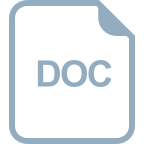
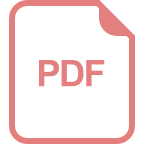
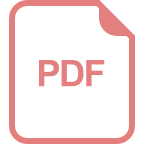
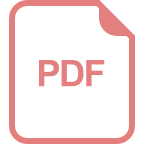
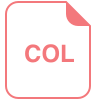








