t1 = threading.Thread(target=run_func, args=(1,)) t2 = threading.Thread(target=run_func, args=(2,)) t3 = threading.Thread(target=run_func, args=(3,)) # ... # 启动线程 t1.start() t2.start() t3.start()显示线程t1,t2,t3中的打印信息
时间: 2024-02-18 16:02:03 浏览: 64
可以在 `run_func` 函数中添加打印线程信息的代码,然后在每个线程中都打印信息。示例代码如下:
```python
import threading
def run_func(thread_id):
print("This is thread t%d" % thread_id)
t1 = threading.Thread(target=run_func, args=(1,))
t2 = threading.Thread(target=run_func, args=(2,))
t3 = threading.Thread(target=run_func, args=(3,))
# 启动线程
t1.start()
t2.start()
t3.start()
```
运行这段代码后,控制台会依次输出线程t1、t2和t3的信息,即:
```
This is thread t1
This is thread t2
This is thread t3
```
相关问题
t1 = threading.Thread(target=run_func, args=(1,)) t2 = threading.Thread(target=run_func, args=(2,)) t3 = threading.Thread(target=run_func, args=(3,)) # ... # 启动线程 t1.start() t2.start() t3.start()显示线程t1中的打印信息
这个需要在 `run_func` 函数中加入打印信息的代码,然后在代码中指定只打印线程t1中的信息。例如,可以在 `run_func` 函数中添加线程编号作为参数,然后在打印时只在编号为1的线程中打印信息。示例代码如下:
```python
import threading
def run_func(thread_id):
if thread_id == 1:
print("This is thread t1")
elif thread_id == 2:
print("This is thread t2")
elif thread_id == 3:
print("This is thread t3")
else:
print("Invalid thread id")
t1 = threading.Thread(target=run_func, args=(1,))
t2 = threading.Thread(target=run_func, args=(2,))
t3 = threading.Thread(target=run_func, args=(3,))
# 启动线程
t1.start()
t2.start()
t3.start()
```
运行这段代码后,只会在控制台中看到线程t1的信息,即:
```
This is thread t1
```
threading.Thread传递参数
可以通过两种方式将参数传递给`threading.Thread`对象:
1. 将参数作为`target`函数的参数传递
```python
import threading
def my_func(arg1, arg2):
# do something with arg1 and arg2
pass
t = threading.Thread(target=my_func, args=(arg1_value, arg2_value))
t.start()
```
2. 将参数作为`Thread`对象的属性传递
```python
import threading
def my_func():
# do something with self.arg1 and self.arg2
pass
t = threading.Thread(target=my_func)
t.arg1 = arg1_value
t.arg2 = arg2_value
t.start()
```
在第二种方式中,参数被保存为`Thread`对象的属性,可以在`target`函数内部通过`self`来访问它们。
相关推荐
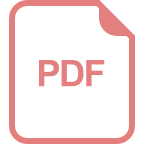
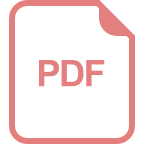














