用C语言怎么判断不同字符串执行不同的运算
时间: 2024-10-16 13:17:10 浏览: 18
在C语言中,你可以通过函数指针或结构体结合动态内存分配来实现根据不同字符串执行不同的运算。这里有一个简单的例子:
1. 函数指针:定义一系列指向运算函数的指针,每个函数对应一种运算。例如,你可以有`add`, `subtract`, `multiply`, `divide`等函数。
```c
#include <stdio.h>
#include <stdlib.h>
void add(const char* str) {
// 实现加法运算
}
void subtract(const char* str) {
// 实现减法运算
}
// ... 其他运算函数
int main() {
void (*op)(const char*) = NULL; // 定义函数指针
scanf("Enter operation: %s", &str); // 用户输入运算符
switch (str[0]) {
case '+':
op = add;
break;
case '-':
op = subtract;
break;
// 添加更多case处理其他运算符
default:
printf("Invalid operator.\n");
return 1;
}
if (op != NULL) {
// 调用对应的函数
op(str);
} else {
printf("No operation provided.\n");
}
return 0;
}
```
2. 结构体和函数指针数组:如果字符串代表的是某种操作的名称,可以将操作作为结构体的一部分,然后存储在数组中。
```c
typedef struct {
const char* name;
void (*func)(const char*);
} Operation;
Operation operations[] = {
{"+", add},
{"-", subtract},
// ... 其他运算结构
};
main() {
char input[256];
scanf("%s", input);
for (size_t i = 0; i < sizeof(operations) / sizeof(Operation); ++i) {
if (!strcmp(input, operations[i].name)) {
operations[i].func(input);
break;
}
}
// ...后续处理
}
```
阅读全文
相关推荐
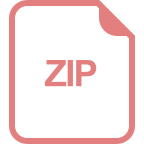
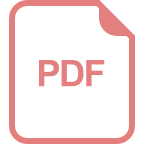
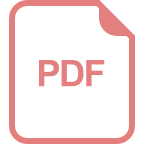
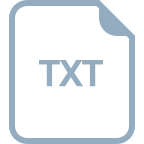
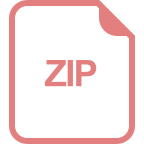
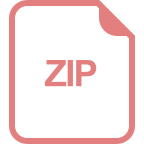
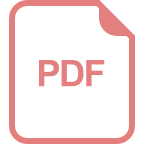
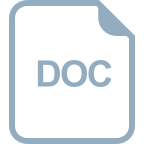
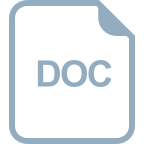
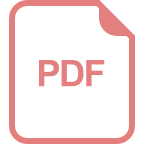
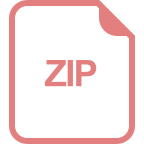
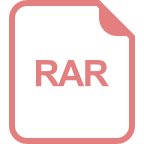
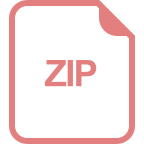
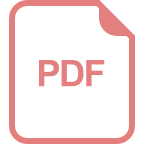
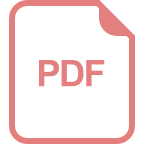
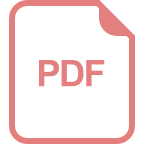
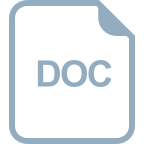
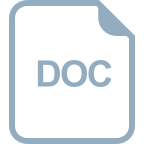
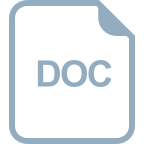