如何将获取到的时间秒数字符串转为数字
时间: 2024-01-29 12:03:38 浏览: 77
要将获取到的时间秒数字符串转换为数字,你可以使用C语言中的库函数`atoi()`或`strtol()`。
以下是使用`atoi()`函数的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char timeStr[] = "12345";
int seconds = atoi(timeStr);
printf("Seconds: %d\n", seconds);
return 0;
}
```
在上述代码中,我们将时间秒数字符串`"12345"`存储在字符数组`timeStr`中。然后,使用`atoi()`函数将该字符串转换为整数,并将结果存储在变量`seconds`中。最后,使用`printf()`函数打印出转换后的秒数。
另外,如果你需要更灵活地处理错误或者处理大于`int`类型范围的秒数,可以使用`strtol()`函数:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char timeStr[] = "12345";
char* endPtr;
long seconds = strtol(timeStr, &endPtr, 10);
if (*endPtr != '\0') {
printf("Invalid input!\n");
return 1;
}
printf("Seconds: %ld\n", seconds);
return 0;
}
```
在这个示例中,我们使用`strtol()`函数将时间秒数字符串转换为长整型。同时,我们还检查了转换后的字符串指针`endPtr`是否到达了字符串的结尾,以判断是否存在非法输入。
阅读全文
相关推荐
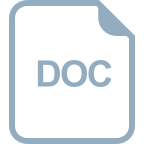
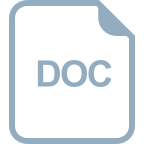
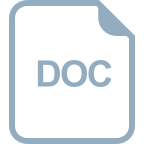
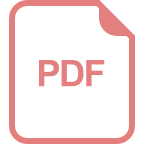
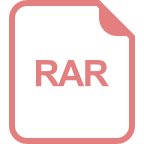










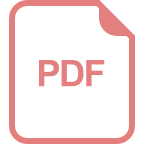


