org.apache.cxf 需要怎么做
时间: 2023-12-11 17:04:04 浏览: 41
您可以按照以下步骤使用 Apache CXF 库:
1. 下载 Apache CXF 库:您可以从 Apache CXF 的官方网站(http://cxf.apache.org/download.html)下载最新版本的 Apache CXF 库。
2. 添加 Apache CXF 库到项目中:下载完 Apache CXF 库后,将它们添加到项目的 Classpath 中。您可以在项目的构建工具(如 Maven 或 Gradle)中添加以下依赖关系:
```xml
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-core</artifactId>
<version>3.4.1</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxws</artifactId>
<version>3.4.1</version>
</dependency>
```
3. 创建服务接口:创建一个 Java 接口,该接口定义您要调用的 Web 服务方法。例如:
```java
import javax.jws.WebMethod;
import javax.jws.WebService;
@WebService
public interface YourServiceInterface {
@WebMethod
String yourMethodName(String yourParameter);
}
```
4. 创建服务类:创建一个 Java 类,该类实现您在第 3 步中创建的接口。例如:
```java
public class YourServiceClass implements YourServiceInterface {
@Override
public String yourMethodName(String yourParameter) {
// 实现您的逻辑
return "Your result";
}
}
```
5. 创建客户端:使用 `JaxWsProxyFactoryBean` 类创建 Web 服务客户端。例如:
```java
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
public class YourClass {
public static void main(String[] args) {
String wsdlUrl = "http://10.9.47.29:8011/ESB/ProxyService/UUM/AccountAndDepartmentService?wsdl";
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setAddress(wsdlUrl);
// 设置接口类和服务类
factory.setServiceClass(YourServiceInterface.class);
factory.setServiceBean(new YourServiceClass());
YourServiceInterface service = (YourServiceInterface) factory.create();
// 调用web service方法
String result = service.yourMethodName(yourParameters);
System.out.println(result);
}
}
```
6. 运行客户端:运行上述代码即可调用您的 Web 服务。
相关推荐
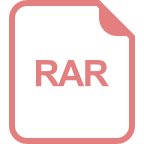
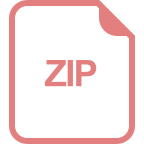














